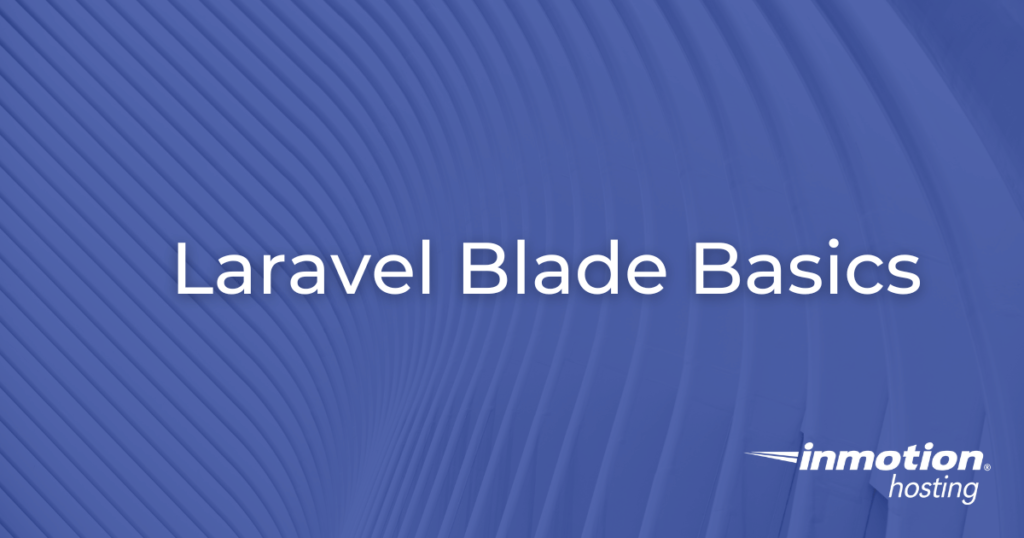
Laravel Blade, Laravel’s native templating engine, stands out for its ability to elegantly integrate PHP with HTML. This synergy simplifies the development of dynamic web interfaces, making Blade a cornerstone of Laravel’s appeal.
In this comprehensive guide, we’ll delve into the fundamentals of Blade, equipping you with the knowledge to craft responsive and interactive web views with ease.
Choose from our Laravel Hosting, VPS Hosting, or Dedicated Servers to host your Laravel applications and websites today!
- What is Blade?
- Setting Up a Blade Template
- Routing to a Blade Template
- Blade Syntax Basics
- Blade Template Inheritance
- The @include Directive
- Laravel Blade Components
- Conclusion
What is Blade?
Blade is a templating engine that comes bundled with Laravel. Unlike traditional PHP templates, Blade templates are compiled into plain PHP code and cached for optimal performance. Blade offers a cleaner and more concise syntax for embedding PHP code in HTML, and it provides a range of helpful directives for common tasks.
Setting Up a Blade Template
Blade templates are identified by the .blade.php
extension and are typically housed in the resources/views
directory. Creating a Blade view is as simple as crafting a new file with this extension. Within this file, you can seamlessly blend HTML and Blade syntax. When it’s time to display a Blade view, Laravel’s view()
function comes into play, rendering the template with any necessary data.
Routing to a Blade Template
Routing in Laravel is the process of directing HTTP requests to specific controllers or closures. To display a Blade template, you first define a route in the routes/web.php
file. For example:
Route::get('/welcome', function () {
return view('welcome');
});
This route uses a closure to return the welcome.blade.php
view when the /welcome
URL is accessed. Laravel’s routing system is incredibly flexible, allowing for the passing of data to views, route parameters, and the use of controllers.
Route::get('/about', function () {
return view('welcome', ['title' => 'Welcome!', 'name' => 'User']);
});
In this example, we are passing an array with title
and name
to the welcome.blade.php
view.
Blade Syntax Basics
Blade simplifies embedding PHP in HTML. For instance, {{ $variable }}
displays a variable, with Blade handling HTML entity escaping.
<! DOCTYPE html>
<html>
<head>
<title>{{ $title }}</title>
</head>
<body>
<h1>Welcome {{ $name }}!<h1>
</body>
</html>
As you can see in this example, we are using the title
and name
variables that were passed to the view.
Control structures like @if
, @foreach
, and @while
offer readable alternatives to PHP tags, enhancing the clarity of your templates.
@if and @endif
@if
and @endif
allow you to conditionally display content based on certain conditions, similar to how you would use if
statements in standard PHP code.
@if($user->'User')
<h1>Welcome Back, User!</h1>
@endif
In this example, the text “Welcome Back, User!” will only be displayed if the $user
variable evaluates to User
.
@foreach and @endforeach
@foreach
and endforeach
are used for looping over arrays or collections, similar to PHP’s foreach
loop.
<ul>
@foreach($users as $user)
<li>{{ $user->name }}</li>
@endforeach
</ul>
In this example, the foreach
loop iterates over a collection of users. For each user in the collection, it creates a list item displaying the user’s name.
@while and @endwhile
@while
and @endwhile
allow you to execute a block of code repeatedly as long as a given condition is true, similar to PHP’s while
loop. They are particularly useful for iterating through data when the number of iterations is not known beforehand.
@php
$count = 0;
@endphp
<ul>
@while($count < count($items))
<li>{{ $items[$count] }}</li>
@php $count++; @endphp
@endwhile
</ul>
In this example, the template creates a list item (<li>
) for each element in the $items
array. The $count
variable is incremented within the loop to eventually break the condition.
Blade Template Inheritance
Blade’s template inheritance feature promotes code reusability. By defining a master layout with common elements, you can extend this layout in other views using @extends
, @section
, and @yield
. This DRY approach streamlines view creation and maintains consistency across your application.
Creating a Master Layout
<!DOCTYPE html>
<html>
<head>
<title>App Name - @yield('title')</title>
</head>
<body>
<header>
<!-- Header content -->
</header>
<div class="container">
@yield('content')
</div>
<footer>
<!-- Footer content -->
</footer>
</body>
</html>
In this layout, @yield('title')
and @yield('content')
are placeholders for content that will be filled by the child views.
Extending the Master Layout in Child Views
Once you have a master layout, you can create individual views that extend this layout. To do this, use the @extends
directive. Within these child views, you define sections (@section
) that correspond to the @yield
directives in the master layout.
@extends('layouts.master')
@section('title', 'Home Page')
@section('content')
<h1>Welcome to Our Application</h1>
<p>This is the home page.</p>
@endsection
In this example, the @extends('layouts.master')
directive tells Blade that this view should inherit the master layout. The @section('title', 'Home Page')
and @section('content')
directives fill in the @yield('title')
and @yield('content')
sections of the master layout, respectively.
The @include Directive
The @include
directive in Laravel’s Blade templating engine is a simple yet powerful feature for including subviews within a Blade view. It promotes modularity by allowing you to break down views into smaller, reusable parts. This approach is particularly useful for elements that are common across multiple pages, such as headers, footers, or navigation bars.
Creating the Subviews
First, we create separate Blade files for the header and footer.
<header>
<h1>My Application</h1>
<nav>
<!-- Navigation links -->
</nav>
</header>
<footer>
<p>© 2023 My Application</p>
</footer>
Including the Subviews in a Main View
Now, we can include these subviews in our main Blade template.
<!DOCTYPE html>
<html>
<head>
<title>Home Page</title>
</head>
<body>
@include('includes.header')
<div class="content">
<p>Welcome to the home page of My Application!</p>
</div>
@include('includes.footer')
</body>
</html>
In this home page template, we use @include('includes.header')
to include the header and @include('includes.footer')
for the footer. These directives pull in the content from the respective header and footer Blade files.
Laravel Blade Components
With the introduction of Blade components in Laravel 7, developers gained a more encapsulated and robust method for building reusable UI elements. These components enhance the modularity of your application’s design, allowing for cleaner and more maintainable code.
Creating and Using a Blade Component
Let’s create a simple Blade component for a button and demonstrate how to use it in a view.
First, we create a new Blade file for the button component.
<button type="{{ $type }}" class="btn {{ $class }}">
{{ $slot }}
</button>
In this component, {{ $type }}
is a variable for the button type (like ‘submit’, ‘button’, etc.), {{ $class }}
allows additional CSS classes to be applied, and {{ $slot }}
is used for the button’s label or content.
To use the component in a Blade view, you use the <x-
tag syntax. Here’s how you can use the button component in a form:
<form action="/submit" method="post">
<!-- Form fields here -->
<x-button type="submit" class="btn-primary">
Submit
</x-button>
</form>
In this example, <x-button type="submit" class="btn-primary">
creates a submit button with the primary button styling. The text between the tags “Submit” is passed into the component and rendered where {{ $slot }}
is in the button component.
Benefits of Using Blade Components
- Encapsulation: Components encapsulate both the structure and logic of UI elements, making them more self-contained and easier to manage.
- Reusability: Components can be reused across different parts of your application, reducing code duplication.
- Customization: Components can accept parameters and content, allowing for flexible customization when reused.
Conclusion
Blade is an indispensable part of the Laravel ecosystem, offering a blend of simplicity and power for web interface development. Its intuitive syntax, combined with Laravel’s robust features, makes Blade an ideal choice for developers seeking to build efficient, maintainable, and interactive web applications.