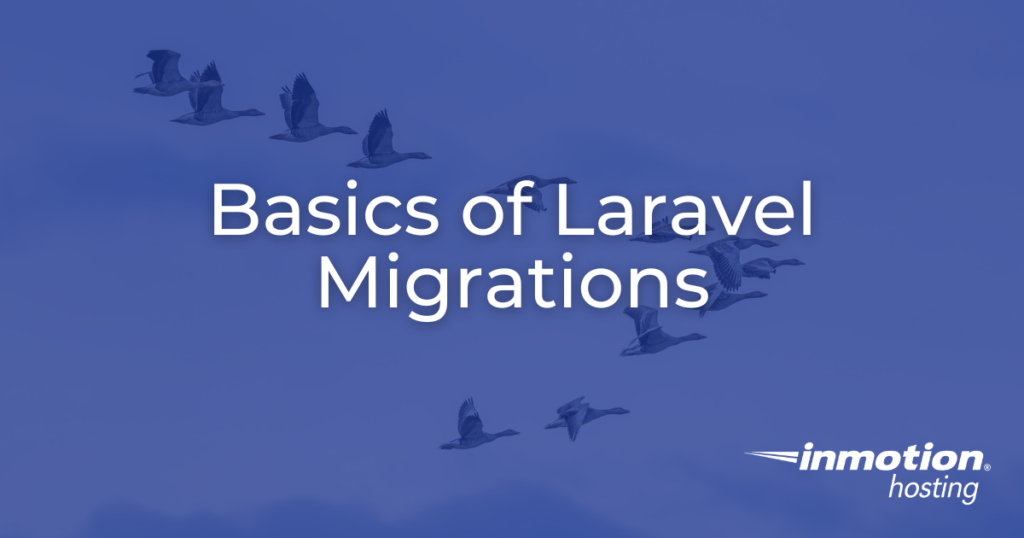
For any web application or website, managing the integrity and consistency of a database schema over time can be a challenge, particularly when working within collaborative team environments or across various deployment stages. Laravel offers a robust feature known as migrations that simplifies this process. Migrations act as version control for your database, enabling developers to modify, share, and track changes to the application’s database schema in a reliable manner. This comprehensive guide introduces the basics of Laravel migrations, providing essential knowledge and practical skills to effectively implement and manage database migrations.
- Understanding Migrations
- Preparing for Migrations
- Creating Migrations
- Running Migrations
- Rolling Back Migrations
- Advanced Migration Operations
- Best Practices for Managing Migrations
- Troubleshooting Common Migration Issues
- Conclusion
Understanding Migrations
Migrations serve as a method for tracking changes to a database schema over time, effectively allowing teams to apply and share these changes systematically. Each migration file in Laravel includes methods that describe how to apply and roll back database changes, thus offering a way to version control your database setup. This capability is crucial for several reasons:
Collaboration and Consistency
Migrations are essential in team-based development environments. They ensure that all members of a team are working with the same database schema without needing to manually adjust database states or share SQL dumps. This streamlined approach not only saves time but also reduces the risk of inconsistencies between development, staging, and production environments.
Simplified Deployment
With migrations, deploying changes to different environments becomes a seamless process. You can push updates to your repository, and migrations can be executed as part of the deployment process to ensure that your database schema matches the application’s requirements automatically. This eliminates the manual effort typically involved in deploying database changes, which can be error-prone and time-consuming.
Easy Reversion
One of the significant advantages of migrations is the ability to quickly revert changes to an earlier database schema version without impacting the current data. This feature is crucial during times when a new schema change leads to unforeseen issues, and a rollback is necessary to maintain the integrity and availability of the application.
Development Efficiency
Migrations speed up the development process by allowing developers to quickly set up their local development environment to match the application’s current schema state. This means new team members or contributors can get up and running quickly, without needing to perform complex database syncs.
Safe and Incremental Schema Evolution
Migrations allow the database schema to evolve incrementally, ensuring that each change is applied in a controlled and staged manner. This incremental approach helps minimize disruptions and detect potential issues early, facilitating smoother transitions as the application scales.
Preparing for Migrations
Prerequisites
Before diving into creating migrations, you must ensure that your Laravel environment is correctly set up:
- Ensure Laravel is installed and properly configured.
- Verify that the database connection settings are correctly specified in the
.env
file. - Confirm that
artisan
, Laravel’s command-line interface, is functioning and accessible for managing migrations.
Migration Files
Migrations are stored within the database/migrations
directory. Files are typically named to include a timestamp of their creation to help Laravel determine the order of execution. This systematic naming convention helps in managing and understanding the flow of database schema evolution.
Creating Migrations
Creating a migration is straightforward with Laravel’s Artisan command-line tool. To create a new migration, you can use the php artisan make:migration
command:
php artisan make:migration create_users_table --create=users
This command generates a new migration file for creating ausers
table. The migration file will be located in thedatabase/migrations
directory, named with a timestamp and the specified namecreate_users_table
.
Structure of a Migration File
A typical migration file contains two primary methods:
up()
: Defines the changes to apply to the database such as creating new tables or modifying existing ones.down()
: Defines how to revert the changes made by theup()
method, essentially rolling back to the previous database schema state.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('first_name');
$table->string('last_name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
Schema::create('password_reset_tokens', function (Blueprint $table) {
$table->string('email')->primary();
$table->string('token');
$table->timestamp('created_at')->nullable();
});
Schema::create('sessions', function (Blueprint $table) {
$table->string('id')->primary();
$table->foreignId('user_id')->nullable()->index();
$table->string('ip_address', 45)->nullable();
$table->text('user_agent')->nullable();
$table->longText('payload');
$table->integer('last_activity')->index();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('users');
Schema::dropIfExists('password_reset_tokens');
Schema::dropIfExists('sessions');
}
};
Example of Defining Schema
Here is how you might define a schema within the up()
method to create a basic users
table:
Schema::create('users', function (Blueprint $table) {
$table->id(); // Auto-incremental primary key
$table->string('name'); // A name column that accepts strings
$table->string('email')->unique(); // A email column that accepts strings that have to be unique
$table->timestamp('email_verified_at')->nullable(); // A created_at and updated_at timestamp column
$table->string('password'); // A password column that accepts strings
});
Running Migrations
To apply your migrations to the database, use the following Artisan command:
php artisan migrate
This command processes all migration files that have not yet been applied, updating the database schema according to the definitions in the up()
methods of the migration files. Laravel smartly keeps track of which migrations have already been executed, so only new or unapplied migrations will run.
Rolling Back Migrations
Laravel provides an efficient way to undo migrations through the following command:
php artisan migrate:rollback
This command rolls back the last batch of migrations, which could include multiple files depending on how they were batched during the migration. For developers needing to completely reset their database schema, the following command can be utilized:
php artisan migrate:reset
Advanced Migration Operations
Laravel’s migration system is not limited to just creating and rolling back tables but also supports complex schema operations. Here are some advanced operations you might need to perform:
Adding Indexes
Indexes can be added to columns to improve the performance of queries involving those columns:
$table->index(['email', 'type']);
Modifying Existing Columns
When you need to change properties of existing columns, Laravel 11 supports these operations natively (previous versions were through the Doctrine DBAL library).
$table->string('email', 191)->change();
Best Practices for Managing Migrations
To ensure that your migrations are easy to manage and understand, consider following these best practices:
- Keep Migrations Specific: Limit each migration file to handling a single specific change. This makes migrations easier to understand and manage.
- Use Descriptive Names: Choose names for your migration files that clearly describe what the migration does. This helps other developers understand the purpose of each migration without needing to read the entire file.
- Commit Regularly: Regularly commit your migration files to your version control system. This keeps your team in sync and allows new team members to set up their development environments quickly by running existing migrations.
Troubleshooting Common Migration Issues
While working with migrations, developers might encounter several common issues. Here are a few troubleshooting tips:
- Duplicate Column Errors: Make sure that your migrations do not attempt to add columns that already exist in the table. Carefully managing migration files and reviewing them before deployment can help avoid such issues.
- Database Connection Errors: Always double-check your database configuration settings in the
.env
file if you encounter connection errors during migration operations. Ensuring that the database server is accessible from your application environment is also crucial.
Conclusion
Migrations are a vital tool for any Laravel developer, providing a robust method for managing database schemas. They facilitate easy collaboration within teams, streamline the deployment process, and enhance the overall reliability of database operations in Laravel applications. By integrating migrations into your development workflow, you can significantly reduce the likelihood of issues related to database schema changes and improve the efficiency of your development processes.
Boost your Laravel apps with our specialized Laravel Hosting. Experience faster speeds for your Laravel applications and websites thanks to NVMe storage, server protection, dedicated resources, and optimization tools.
99.99% Uptime
Free SSL
Dedicated IP Address
Developer Tools