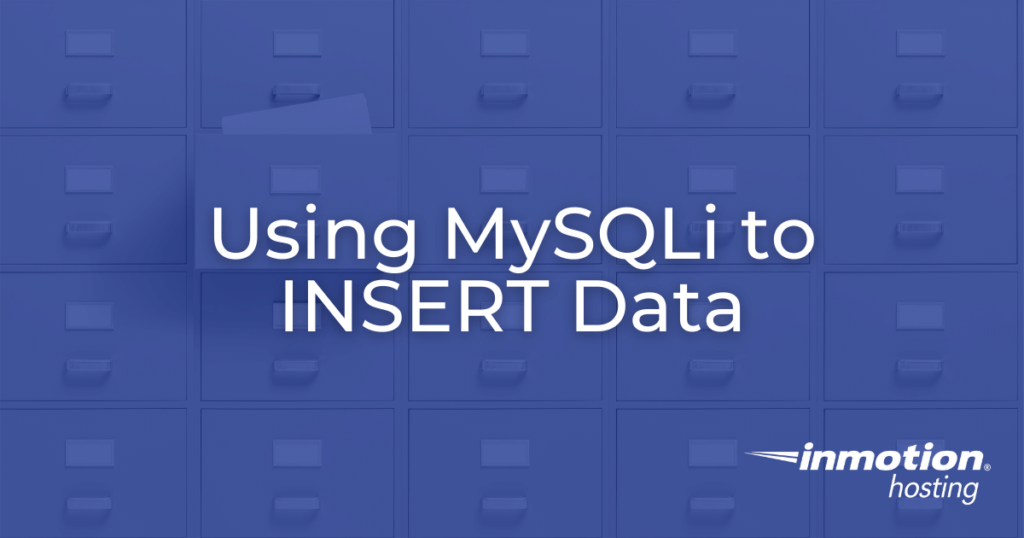
Since newer versions of PHP no longer support the ability to insert data into a database using PHP extensions, users will need to make use of an extension such as MySQLi to insert data into their databases. Using MySQLi to insert data can be done directly in the command line or via PHP script. In this article we will discuss using MySQLi to insert data into MySQL databases.
Using MySQLi to INSERT Data
- First, ensure that your database has been created and can be accessed.
- Next, connect to your database server via SSH. Please note that this will require the use of command-line operations. It is only recommended to perform these steps if you are comfortable using command-line interfaces.
- Once you’ve connected to your database server you will want to log into MySQL.
- Once logged in, you can use the mysql prompt to execute the following query and insert data into your database.
$sql = “INSERT INTO data_product1 (size, color, price) VALUES (‘M’, ‘Blue’, ‘39.99’)”;
- In our example, data_product represents the database table being modified. The size, color, and price all represent separate columns in the database structure. The values represent the data being stored, in this case the size, color, and price of the product in question. For a typical eCommerce website, a database will contain thousands of tables storing a variety of data ranging from contact information to product descriptions and specifications.
Creating a PHP script to INSERT Data using MySQLi
While it is not possible to use PHP extensions to insert data, you can still write a PHP script that uses the MySQLi extension to insert the data.
The first portion of the script will involve connecting to the database. The dbhost is the hostname of the database server, usually localhost. The dbuser is the database username, dbpass is the password for the database user, and the dbname is the name of the database itself:
<html> <head> <title>Adding Product Data</title> </head> <body> <?php $dbhost = 'localhost'; $dbuser = 'root'; $dbpass = 'securepassword123'; $dbname = 'eCommercedata'; $mysqli = new mysqli($dbhost, $dbuser, $dbpass, $dbname);
The next portion of the script will contain the INSERT query with the same information we used in the previous section.
$sql = "INSERT INTO data_product1 ". "(size, color, price) "."VALUES ". "('M','Blue','39.99$')"; ?> </body> </html>
Save this file as mysqli_insertexample.php for use with your web server.
Congratulations, you now know how to insert data using MySQLi!
admins should consider updating this article. mysql has been deprecated. instead use mysqli (or PDO). if you need to see errors, turn on display_errors in the multiphp ini editor for your domain (being sure to apply that). if you moved in an old database and are trying to continue to insert data, know that doing an insert will fail if any fields not being populated in that new row have a default of ‘none’, which you will have change to a default of NULL for that column in phpmyadmin – or your table will not accept data.
Thanks for this info. We’ll look into updating this.
The information in this article is out of date. I would not suggest using this tutorial at all (and it is alarming that it is still being shown by a hosting company).
PHP has completely stopped supporting the `mysql_real_escape_string` function in releases starting in 2015. Please look at showing people how to use PDO or MySQLi for MySQL database connections and the importance of prepared statements in stopping injection attacks. If the query is not static, you should be using a prepared statement.
Thanks for your feedback. Your contribution to the community is especially appreciated.
Thanks sir,
This tutorial was really pretty and userful to create a connection of database and known the insert query in php.It works awesome.
Thanks again.,
Well it only does it when I have your code called on the main index.php page.
Now wondering how I could have this POST within it’s own DIV on the main index page ALONG side of the SELECT function, so people’s comments show up right beside the input, immediitely.
Any input would be great, much appreciated.
When the issues were occuring, it looks like when the index page refreshes it wants to submit the POST function twice, and my auto-increment and private key are correct and treat it like 2 php submits. (Not a DB issue)
This was very easy to follow and informative. A+ job standing out from the many PHP walk-throughs out there. Will share when I come accross other forums when it relates. Thank you.
Hey,
Have you ran into the issue where the POST is inserting the values twice?
It shows two entries: the increment is working, but its the same name, comments, and exact timestamp?
Thanks.
super
Shaik, I’m glad we were able to help!
Hi
My name is Larry
I tried the original and am getting errors like
ERROR INSERT Into ‘dnrbmus_me’. ‘col'(‘name’,’phone’,’cell’,’email’)
VALUES(‘e’,’1′,’6′,’3′);’
When looking at my do nothing gets in putted .
Since this is just one article in a full series, ensure you have followed the previous steps for this guide to work.
Also here is a helpful link to a helpful guide on the INSERT INTO statement.
Thank you,
John-Paul
Thank you,
John-Paul
Sham, I’m glad that we were able to assist you!
i’m tryin to run this code but it contined to give me fatal erro i need srioue hel just a starting
<?php
$name = $_POST[‘name’];
$email = $_POST[’email’];
$password = $_POST[‘password’];
if($name && $email && $password){
mysqli_connect(“localhost”, “root”, “”) or die(“we couldn’t connect”);
mysql_Select_db(“testsite”);
mysql_query(“INSERT INTO users(name,email,password) VALUES(‘$name’,’$email’,’$password’)”);
$registered = mysqli_affected_rows();
echo “$registered was inserted”;
}else{
“you have to compelet the form!”;
}
What is the full “fatal error” message you are getting?
Thank you,
John-Paul
I read in a few posts here that people did not get an error message but still the database did not get populated. As suggested I let my site echo $query and ran the result in the phpMyAdmin>SQL tab. I got the error message saying “id cannot be empty”. Turned out I forgot to check the A_I (auto increment) for the id column. Spent quite some time searching so if you have similar problems, please check this one first : ).
I am showing no errors on my webpage or in my web servers error log but still nothing gets inserted into the database. Obviously my work has reached a stand still. I would post my code, but i cant attach files and copying and pasting lends itself to indentation errors. I can add more info if requested.
Thanks,
Luke D.
Hello Luke D.,
Sorry for the problems with inserting data into the database. If you are using the code directly as it is shown above, then it should be working. If you are using a variant on the code, then we unfortunately cannot assist as we do not provide coding support. Try using the code above. If you’re using something different and still need assistance, then you may want consider posting in a programming/coding forum, or consulting with a developer.
If you have any further questions or comments, please let us know.
Regards,
Arnel C.
I get this error message when I try to post a comment from page1.php?id=1
Could not connect: “Access denied for user ‘my use [email protected]’ (using password: YES)
Exactly what goes in this line of code?
Hello Bob,
Sorry for the problems with connecting to the database. The database error appears to try to connect to a Godaddy server. If you’re trying to connect a database outside of your hosting service, then you may need to make a remote connection in cPanel (at least this is how we do it at InMotion). The information that you supplied indicates the connection information if you are hosting your website with InMotion and connecting to the MySQL server that comes with your account. If you’re trying to connect to the database using a different host, then you may need to look at their requirements as they may be different. If you’re using the connection information as per the code above:
Make sure that you did not forget the database identification line as well.
I hope this helps to answer your question, please let us know if you require any further assistance.
Regards,
Arnel C.
Hi, I’m very new to coding and things like this, and I’m having trouble. I got to the first step of this page (inserting a test comment), and I left the ‘id’ field blank as shown. When I inserted it, however, it gave me this:
1 row inserted.
Warning: #1366 Incorrect integer value: ” for column ‘id’ at row 1
Here is the insert command I was given:
INSERT INTO `bawrestore`.`comments` (`id`, `name`, `email`, `comment`, `timestamp`, `articleid`) VALUES (”, ‘Casey White’, ‘[email protected]’, ‘Comment’, CURRENT_TIMESTAMP, ‘1’);
(Notice how in ‘VALUES’, the ‘id’ spot is just ” instead of ‘NULL’ like in the example image)
I don’t know what I should do to fix this, and I would like some help, please.
Thanks!
If you have a field for id and do not fill it, it will not be NULL, but basically a blank space. If the id is a required field you will need to compensate for that in the code.
Pleas help me cuz i dont kow why after submission, it echos submitted sucessfully but i cant see anything in my db and also throws up this error message
Message Submitted Successfully”; mysql_close($con); ?>
<?
if( $_POST )
{
$con = mysql_connect(“localhost”,”ailogeng_aiogdb”,”hotdog1470″);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“ailogeng_aiogdb”, $con);
$users_name = $_POST[‘userName’];
$users_email = $_POST[‘userEmail’];
$users_phone = $_POST[‘userPhone’];
$users_message = $_POST[‘userMsg’];
$users_name = mysql_real_escape_string($users_name);
$users_email = mysql_real_escape_string($users_email);
$users_website = mysql_real_escape_string($users_phone);
$users_message = mysql_real_escape_string($users_message);
$articleid = $_GET[‘id’];
if( ! is_numeric($articleid) )
die(‘invalid article id’);
$query = “INSERT INTO `ailogeng_aiogdb`.`aigeng` (`userName`, `userEmail`, `userPhone`, `userMsg`) VALUES (”, ”, ”, ”);”;
mysql_query($query);
echo “<h2>Message Submitted Successfully</h2>”;
mysql_close($con);
?>
Hello victor,
Thank you for contacting us. This is not the typical behavior for this script. I recommend reviewing your syntax to ensure you have not missed a ‘;’ or code.
Can you provide a link to the code for us to test and replicate further?
Thank you,
John-Paul
Nice
I have been troubleshooting this problem independently for hours now and after looking at the initial “John Smith” INSERT code that we save to a text file I realized that the ‘comments’ part was lower-case while my ‘Comments’ database is capitalized. I hope this helps others because I was getting a little stressed out!! lol
hi i just put this code into my blog (that i have not yet posted to the internet) and i tested it out, but when i hit submit it said” thank you for your post ; mysql_close($con);}?>” how doi fix it?
Hello Madi,
The code is to help you insert data from the web. Did you use all of the code verbatim? What code did you use exactly? The “thank you for your post” is part of the code. You may need to adapt it for your own use.
If you have any further questions, please let us know.
Kindest regards,
Arnel C.
<?php
include(“new/config.php”);
?>
<?php
if (isset($_Post[‘btn’])){
$username=$Post[‘UName’];
$password=$Post[‘pass’];
$Gender=$Post[‘Gender’];
$Name=$Files[‘img’][‘name’];
$Size=$Files[‘img’][‘size’];
$Temp=$Files[‘img’][‘tmp_name’];
$Type=$Files[‘img’][‘type’];
if(empty($username)||empty($password)||empty($Gender)){
echo “Fill the Required Fields”;
}
else{
if($Size<=0){
$error= “<h1>Fill the Required Fields</h1>”;
}
else
{
$dir=”img/”;
move_uploaded_file($Temp,$dir.$Name);
$query=”INSERT INTO login values(”,’$username’,’$password’,’$Name’,’$Gender’)”;
$sql=mysqli_query($con,$query);
$success = “<p>Record has been Submitted</p>”;
}
}
}
?>
<html>
<style>
*{
margin:0;
padding:0;
}
section{
width:100%;
background:#333;
padding:13% 0 14% 0;
}
.login-wrapper{
width:70%;
margin:0 auto;
}
.control{
width:50%;
margin:0 auto;
padding:1% 0 1% 4%;
box-sizing:border-box;
}
.control .form{
width:90%;
padding:1.5%;
font-size:120%;
border:1px solid #fff;
background:black;
color:white;
transition:all .3s ease;
}
.form:focus{
border:1px solid orange;
color:white;
-webkit-box-shadow: inset 7px 8px 71px -39px orange;
-moz-box-shadow: inset 7px 8px 71px -39px orange;
box-shadow: inset 7px 8px 71px -39px orange;
}
.control .browse{
width:50%;
border:1px solid #fff;
background:black;
color:white;
}
.control select{
border:1px solid orange;
width:35%;
padding:1% 0 1% 0;
}
.control option{
width:100%;
padding:1% 0 1% 0;
}
.control .button{
width:35%;
color:white;
padding:1% 0 1% 0;
background:black;
border:1px solid #fff;
transition:all .8s ease;
cursor:pointer;
}
.button:hover{
border:1px solid orange;
color:orange;
}
label{
color:white;
display:block;
font-size:110%;
padding:1% 0 1% 0;
font-family:calibri;
}
section h1{
text-align:center;
color:white;
font-family:candara;
font-size:200%;
}
</style>
<section>
<h1>Sign Up & enjoy</h1>
<?php if (isset($error)) {echo $error;}?>
<?php if (isset($success)) {echo $success;}?>
<div class=”login-wrapper”>
<form method=”post” action=”” enctype=”multipart/form-data”>
<div class=”control”>
<label>User Name</label>
<input class=”form” type=”text” name=”UName”/>
</div>
<div class=”control”>
<label>Password</label>
<input class=”form” type=”password” name=”pass”/>
</div>
<div class=”control”>
<label>User Photo</label>
<input class=”browse” type=”file” name=”img”/>
</div>
<div class=”control”>
<label>Gender</label>
<select name=”Gender”>
<option>Male</option>
<option>Female</option>
</select>
</div>
<div class=”control”>
<input class=”button” type=”submit” name=”btn” value=”submit”/>
</div>
</form>
</div>
</section>
</html>
Hello,
I’m sorry for the problem you may having with the code. We unfortunately do not provide coding support. If you are using the code we provide specifically and it’s not working or if the issue is related to a server-side error then we can investigate the problem in more depth. You may want to consider consulting with a developer/programmer to review your code, or you may utilize a forum that specializes in troubleshooting PHP code. Apologies that we cannot provide any direct assistance on this issue.
If you have any further questions or comments, please let us know.
Regards,
Arnel C.
my code does not work 🙂 I am able to process it but just does not update the db…
<?php
if (isset($_POST[‘submit’])){
$dbhost =”localhost”;
$dbuser =”root”;
$dbpass = “”;
$dbname = “hps”;
// Create a database connection
$connection = mysqli_connect($dbhost, $dbuser, $dbpass, $dbname);
// Test if connection occured
if(mysqli_connect_errno()){
die(“Database connection failed: “.
mysqli_connect_errno() .
“(” .mysqli_connect_errno() .”)”
);
}
//mysql_select_db(“hps”, $connection);
$firstname =$_Post[‘firstname’];
$lastname =$_Post[‘lastname’];
$homebreak =$_Post[‘homebreak’];
$favbreak =$_Post[‘favbreak’];
$foot =$_Post[‘foot’];
$phone =$_Post[‘phone’];
$email =$_Post[’email’];
$passwd =$_Post[‘passwd’];
$repeatpasswd =$_Post[‘repeatpasswd’];
$userphoto =$_Post[‘userphoto’];
$sql = “INSERT INTO surfers (Firstname, Lastname, Homebreak, FavBreak, Surfingstyle, ContactPhone, Email, Password, Salt, SurferPhoto) VALUES (‘$firstname’, ‘$lastname’, ‘$homebreak’, ‘$favbreak’,’$foot’, ‘$phone’, ‘$email’, ‘$passwd’, ‘$repeatpasswd’, ‘$userphoto’)”;
if (!mysqli_query($connection, $sql)) {
die(‘error inserting new record’);
}
$newrecord = “1 record added to the db”;
}
?>
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”utf-8″> <!– specify utf-8 as the character set – can be viewed in multiple languages –>
<meta http-equiv=”X-UA-Compatible” content=”IE=edge”> <!– tell microsoft browsers to use the ‘edge’ version of IE –>
<meta name=”viewport” content=”width=device-width, initial-scale=1″> <!– on mobiles page will display for the mobile’s size not desktop size; no zoom when 1st shown –>
<!– The above 3 meta tags *must* come first in the head; any other head content must come *after* these tags –>
<meta name=”description” content=”High Performance Surf – surf lesson specialists, surf comps competitions bookings private lessons public lessons”> <!– the description will be picked up by search engines and displayed – make it meaningful but directly about your key website purpose – not waffling on –>
<title>High Performance Surf | Homepage</title>
<!– Bootstrap core CSS –>
<link href=”../css/bootstrap.css” rel=”stylesheet”>
<!– HTML5 shim and Respond.js for IE8 support of HTML5 elements and media queries –>
<!–[if lt IE 9]>
<script src=”https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js”></script>
<script src=”https://oss.maxcdn.com/respond/1.4.2/respond.min.js”></script>
<![endif]–>
</head>
<body>
<?php include “../includes/nav-menu.php” ?>
<?php include “../includes/header.php” ?>
<!– Begin page content –>
<div class=”container-fluid”>
<div class=”col-md-2″></div>
<div class=”col-md-6″>Already registered? Just <a href=”#”>login</a> then!<br />
<h1 class=”page-header”>Register with High Performance Surf</h1></div>
<div class=”col-md-4″></div>
</div>
<div class=”container-fluid”>
<div class=”row”>
<div class=”col-md-2″></div>
<div class=”col-md-6″>
<form enctype=”multipart/form-data” method=”post” action=”register.php” role=”form”>
<div class=”form-group”>
<label for=”firstname”>First Name</label>
<input type=”text” class=”form-control” id=”firstname” name=”firstname” required placeholder=”First name”>
</div>
<div class=”form-group”>
<label for=”lastname”>Last Name</label>
<input type=”text” class=”form-control” id=”lastname” name=”lastname” required placeholder=”Last name”>
</div>
<div class=”form-group”>
<label for=”homebreak”>Home break</label>
<input type=”text” class=”form-control” id=”homebreak” name=”homebreak” placeholder=”Where would we mostly likely find you carving out some waves?”>
</div>
<div class=”form-group”>
<label for=”favbreak”>Favourite break</label>
<input type=”text” class=”form-control” id=”favbreak” name=”favbreak” placeholder=”Where’s your fav break?”>
</div>
<div class=”form-group”>
<label for=”favbreak”>Style:</label>
<input type=”radio” id=”foot” name=”foot” value=”regular”> Regular Foot <input type=”radio” id=”foot” name=”foot” value=”goofy”> Goofy Foot
</div>
<div class=”form-group”>
<label for=”phone”>Contact phone</label>
<input type=”text” class=”form-control” id=”phone” name=”phone” placeholder=”Used when comps are shifted”>
</div>
<div class=”form-group”>
<label for=”email”>Email address</label>
<input type=”email” class=”form-control” id=”email” name=”email” required placeholder=”Used for emails and to log in with”>
</div>
<div class=”form-group”>
<label for=”passwd”>Password</label>
<input type=”password” class=”form-control” id=”passwd” name=”passwd” required placeholder=”Used when logging in”>
</div>
<div class=”form-group”>
<label for=”repeatpasswd”>Repeat Password (will be checked in a later unit)</label>
<input type=”password” class=”form-control” id=”repeatpasswd” name=”repeatpasswd” required placeholder=”Re-enter password”>
</div>
<div class=”form-group”>
<label for=”userphoto”>Photo – upload one of you or of you surfing</label> <span class=”help-block”>(A .jpg or .png image viewable by public)</span>
<input type=”file” id=”userphoto” name=”userphoto”>
</div>
<button type=”submit” class=”btn btn-default”>Submit</button>
</form>
</div>
<div class=”col-md-4″></div>
</div>
<!– Add some space –>
<div class=”container”>
<br />
</div>
<!– Bootstrap core JavaScript
================================================== –>
<?php include “../includes/footer.php” ?>
<!– Placed at the end of the document so the pages load faster –>
<script src=”https://ajax.googleapis.com/ajax/libs/jquery/1.11.3/jquery.min.js”></script>
<script src=”../js/bootstrap.min.js”></script>
<?php
echo $newrecord
?>
</body>
</html>
Hello,
Unfortunately we are not able to troubleshoot custom code. However, to help you along you will want to have your code echo out the full Update query, complete with variable values. Take the query and try to run it in a clone of your database to see if it works. If not, it should give you a descriptive error that can help you correct it.
Kindest Regards,
Scott M
Hello,
Thanks for the question about adding your mysql query across multiple pages (and multiple times within a single page). Unfortunately, we do not provide coding support of this nature here. You may want to check out this post in a forum for developing. We do apologize that we can provide a direct answer to your programming questions.
If you have any further questions or comments, please let us know.
Regards,
Arnel C.
Im having the same issue. This is my formcode:
<form method=’post’>
NAME: <input type=’text’ name=’name’ id=’name’ /><br />
Email: <input type=’text’ name=’email’ id=’email’ /><br />
Website: <input type=’text’ name=’website’ id=’website’ /><br />
Comment:<br />
<textarea name=’comment’ id=’comment’></textarea><br />
<input type=’hidden’ name=’articleid’ id=’articleid’ value='<? echo $_GET[“id”]; ?>’ />
<input type=’submit’ value=’Submit’ />
</form>
this is my manage_comments code:
<?
if( $_POST )
{
$con = mysql_connect(“localhost”,”saifi_myuser”,”Firefox1″);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“saifi_mysite”, $con);
$users_name = $_POST[‘name’];
$users_email = $_POST[’email’];
$users_website = $_POST[‘website’];
$users_comment = $_POST[‘comment’];
$users_name = mysql_real_escape_string($users_name);
$users_email = mysql_real_escape_string($users_email);
$users_website = mysql_real_escape_string($users_website);
$users_comment = mysql_real_escape_string($users_comment);
$articleid = $_GET[‘id’];
if( ! is_numeric($articleid) )
die(‘invalid article id’);
$query = “
INSERT INTO `saifi_mysite`.`comments` (`id`, `name`, `email`, `website`,
`comment`, `timestamp`, `articleid`) VALUES (NULL, ‘$users_name’,
‘$users_email’, ‘$users_website’, ‘$users_comment’,
CURRENT_TIMESTAMP, ‘$articleid’);”;
mysql_query($query);
echo “<h2>Thank you for your Comment!</h2>”;
mysql_close($con);
}
?>
page 1:
<? include(“manage_comments.php”); ?>
<h1>This is page1.php</h1>
<div><a href=’page2.php?id=2′>Click here</a> to go to page2.php</div>
<div style=’margin:20px; width:100px; height:100px; background:blue;’></div>
<? include(“formcode.php”); ?>
page 2:
<? include(“manage_comments.php”); ?>
<h1>This is page2.php</h1>
<div><a href=’page1.php?id=1′>Click here</a> to go to page1.php</div>
<div style=’margin:20px; width:100px; height:100px; background:orange’></div>
<? include(“formcode.php”); ?>
Once hitting “submit” the page displays “Thank you for your comment” however nothing is sent to the database.
Hello Saf,
Apologies with the problems with your code. Can you tell us what is happening when you try to use your code? Are you getting a particular error message? Are you using the same code as what is provided in the article above? We do not provide programming support as it is beyond the scope of our support. If you are using something different from the provided code in the article, then you may want to consult with a developer for the issue. We can look at errors and help to point you in helpful direction for issues. If you have any further questions or comments, please let us know.
Regards,
Arnel C.
please can you help me i don’t know were is the error !!!
Not inserted record in mysql. Output shows only error.
<?php
$con = mysql_connect(“localhost”,”root”,””);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“nimswp”, $con);
$con = mysql_connect(“localhost”,”root”,””);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“nimswp”, $con);
// Insert data to database……..
if( isset( $_POST[‘submit’] ) || $_POST[‘submit1′] ==’submit’){
$sql_insert_emp = ‘INSERT INTO nims_wp_user_login(
nism_employe_code,
nims_employe_surname,
nims_employe_fullname,
nims_wp_user_password,
nims_wp_user_email,
nims_employe_mob_no,
nims_employe_email,
nims_wp_user_type
)
VALUES(
‘.$_POST[$nism_employe_code].’,
‘.$_POST[$nims_employe_surname].’,
‘.$_POST[$nims_employe_fullname].’,
‘.$_POST[$nims_wp_user_password].’,
‘.$_POST[$nims_wp_user_email].’,
‘.$_POST[$nims_employe_mob_no].’,
‘.$_POST[$nims_employe_email].’,
‘.$_POST[$nims_wp_user_type].’)’;
if(!$result = mysql_connect($sql_insert_emp, $con)){
echo “Error.”;
}else{
echo “Record Inserted”;
}
?>
Hello laxmirao,
Thank you for contacting us. What is the full error you are receiving?
Are you following our full series on Using PHP to create dynamic pages?
Thank you,
John-Paul
okeey but what is a Support Email ?
Hello Ayoub,
You can submit a ticket to our live technical support team through the AMP interface.
Best Regards,
TJ Edens
the data does not fit into the mysql database
If you send the file to import into the Support Department we can help you get it imported.
please can you help me i don’t know were is the error !!!
<?
if(isset($_POST[‘formexe’]))
{
$con = mysql_connect(“localhost”,”root”,””);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“java”, $con);
$users_Prenom = $_POST[‘Prenom’];
$users_Nom = $_POST[‘Nom’];
$users_Profession = $_POST[‘Profession’];
$users_CIN = $_POST[‘CIN’];
$users_TEL = $_POST[‘TEL’];
$users_Email = $_POST[‘Email’];
$users_apt = $_POST[‘apt’];
$users_dpt = $_POST[‘dpt’];
$users_dpt1 = $_POST[‘dpt1’];
$users_act = $_POST[‘act’];
$users_cia = $_POST[‘cia’];
$users_ef = $_POST[‘ef’];
$users_asso = $_POST[‘asso’];
$users_Prenom = mysql_real_escape_string($users_Prenom);
$users_Nom = mysql_real_escape_string($users_Nom);
$users_Profession = mysql_real_escape_string($users_Profession);
$users_CIN = mysql_real_escape_string($users_CIN);
$users_TEL = mysql_real_escape_string($users_TEL);
$users_Email = mysql_real_escape_string($users_Email);
$users_apt = mysql_real_escape_string($users_apt);
$users_dpt = mysql_real_escape_string($users_dpt);
$users_dpt1 = mysql_real_escape_string($users_dpt1);
$users_act = mysql_real_escape_string($users_act);
$users_cia = mysql_real_escape_string($users_cia);
$users_ef = mysql_real_escape_string($users_ef);
$users_asso = mysql_real_escape_string($users_asso);
$userid = $_GET[‘id’];
if( ! is_numeric($userid) );
$query = “
INSERT INTO `java`.`users` (`id`, `Prenom`, `Nom`, `Profession`,
`CIN`, `TEL`, `Email`, `apt`, `dpt`, `dpt1`, `act`, `cia`, `ef`, `asso`) VALUES (NULL, ‘$users_Prenom’,
‘$users_Nom’, ‘$users_Profession’, ‘$users_CIN’, ‘$users_TEL’, ‘$users_Email’, ‘$users_apt’, ‘$users_dpt’, ‘$users_dpt1’, ‘$users_act’, ‘$users_cia’, ‘$users_ef’, ‘$users_asso’,
‘$userid’);”;
mysql_query($query);
mysql_close($con);
}
?>
What error message are you getting? We’ll need it to help you trouble shoot the issue.
Hey, I tried this snip, but the database is still empty. Help me out..
My form consists of username and email.. So the database..
<?
if( $_POST )
{
$con = mysql_connect(“localhost”,”root”,””);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“users”, $con);
$users_name = $_POST[‘user’];
$users_email = $_POST[‘mail’];
$users_name = mysql_real_escape_string($users_name);
$users_email = mysql_real_escape_string($users_email);
$query = “
INSERT INTO `users`.`userinfo` (`UserName`, `Email`) VALUES (‘$users_name’,
‘$users_email’);”;
mysql_query($query);
echo “<h2>Thank you for your Comment!</h2>”;
mysql_close($con);
}
?>
Hello Karan,
Are you getting any errors? What happens when you try to test the query by itself in phpMyAdmin?
Kindest Regards,
Scott M
thank you for this, Im literally brand new to PHP (less than a week of using it) and you saved me from a terrible headache. Took a day but now i can move forward in what im learning.
Fantastic, thank you.
The trick that helped me was to get the mysql insert code from the database after inserting the dtest record.
I applied this to a csv import file for multiple records, where we only want to insert 3 fields with data per record.
The code may help others, here is the example file import-csv-to-server.php-
<?php
// Open database with connect file
include_once(‘connect.php’);
// Open CSV file for multiple data records to insert
$fo = fopen(‘importfile.csv’, “r”);
while (($data = fgetcsv($fo, 20000, “,”)) !== FALSE)
{
$surname = $data[0];
$firstname = $data[1];
$firm = $data[2];
$query = “INSERT INTO `[database-name]` (`did`, `password`, `surname`, `firstname`, `initials`, `title`, `firm`, `businessdescription`, `credentials`, `email`, `telephone`, `mobile`, `postal1`, `postal2`, `postal3`, `postal4`, `postal5`, `country`, `principle1`, `principle2`, `yearstrust`, `numtrust`, `assets`, `membertype`, `subfund`, `memdisc`, `web`, `profession`, `listwebsite`, `registered_trustee`, `active`, `paymentamount`, `Timestamp`, `firstreceived`, `regfirstreceived`, `regnumber`, `freemembership`)
VALUES
(NULL, ‘Tg7nzt’, ‘$surname’, ‘$firstname’, ”, NULL, ‘$firm’, ”, NULL, NULL, ”, NULL, NULL, NULL, NULL, ”, ”, ‘New Zealand’, NULL, NULL, ‘1’, ‘0’, ‘0’, ”, NULL, ”, NULL, NULL, ‘N’, ‘N’, ‘Y’, ‘N’, CURRENT_TIMESTAMP, ”, ”, ‘0’, ”)”;
mysql_query($query);
}
echo finished;
?>
Here is the example of the CSV data file import.csv
rec1-1,rec1-2,rec1-3,
rec2-1,rec2-2,rec2-3,
rec3-1,rec3-2,rec3-3,
Upload bothe files to your server then run www.[sitename]/import-csv-to-server.php
i m trying to submit data by clicking only submit button in a web service but it submit data also by enter so dive the olution for that
Hello Wasim,
Sorry for the problem with the code. We unfortunately do not provide coding support. However, we did take a look at what you provided and it seems to be okay – nothing jumps out as being the “problem”. We’re not sure if you’re on a shared server or dedicated server – remember that we typically only provide support for hosting services.
If you’re NOT obfuscating the name of the database and the user name, then that’s the first place to start. User names for our database connections are not formatted that way – you would need to use a user assigned to the database. We do suggest that you step through your code, and if something is not appearing (per your echo’s), then review that part of the code. For example, if you’re not getting anything from your first 2 echos, then something’s wrong with that loop. We didn’t think that the database select was required either, as you specify the connection in $conn.
I hope this helps to answer your question, please let us know if you require any further assistance.
Regards,
Arnel C.
Hello umesh,
Thank you for contacting us. There are many solutions, and they will differ based on how your specific form is coded.
I found a possible solution via online search, here is a possible way to Disable Enter or Return key in PHP.
Thank you,
John-Paul
A.o.A
Hi everyone,
i have created a form in html and php.
i have created the same in database.
i entered the name and email in htm file.
but php not display any result or error.
also the data not inserted in database.
Code are as follows:
Please help:
htm code:
<html>
<head>
<title>Form1</title>
</head>
<body>
<form method=”POST” action=”Form1.php”>
Full Name:<br>
<input type=”text” name=”name”><br><br>
Email:<br>
<input type=”email” name=”email”><br><br>
<input type=”submit” name=”submit” value=”submit”>
</form>
</body>
</html>
PHP Code:
<html>
<head>
<title>Form1</title>
</head>
<body>
<?php
$conn=mysql_connect(“localhost”, “root”, “”, “db1”) or die (“not connected”);
mysql_select_db(“db1”);
if (isset ($_post[“submit”])) {
$name=$_POST[“name”];
$email=$_POST[“email”];
echo $_POST[“name”] . “<br>”;
echo $_POST[“email”] . “<br>”;
$sql=”INSERT INTO form1 (Full name, Email) VALUES ($name, $email)”;
if (mysql_query($sql, $conn)) {
echo “All is fine”;
}
else {
echo “Problem not found”;
}
/*$a=mysql_query($sql, $conn);
if (!$a) {
echo “Not Entered….”;
}
else{
echo “Data Entered….”;
}*/
}
?>
</body>
</html>
Hello Ali,
Here is a StackOverflow.com thread that has everything provided on what you would need to do.
Best Regards,
TJ Edens
am hosting an asp.net website but it seems am facing 500-server internal error.
this is the code in my web.config. i really need help. can anyone help me?
<?xml version=”1.0″?>
<!–
For more information on how to configure your ASP.NET application, please visit
https://go.microsoft.com/fwlink/?LinkId=169433
–>
<configuration>
<connectionStrings>
<add name=”AssociationDBConnectionString”
connectionString=”Data Source=.\SQLEXPRESS;AttachDbFilename="C:\Users\ARNOLDSCHWAZZY\Documents\Visual Studio 2010\WebSites\AssociationWebSite\App_Data\CommentDB.mdf";Integrated Security=True;Connect Timeout=30;User Instance=True”
providerName=”System.Data.SqlClient” />
</connectionStrings>
<system.web>
<compilation debug=”true” targetFramework=”4.0″/>
</system.web>
</configuration>
Hello Arnold,
Unfortunately all our servers are Linux based and we are not able to work with or troubleshoot Windows Only issues.
Kindest Regards,
Scott M
I have encountered some errors while registering new doctor but the data is added to the database.The errors encountered are,
Warning: mysql_fetch_array() expects parameter 1 to be resource, boolean given in C:\xampp\htdocs\clinicosight\doctors.php on line 7
Warning: fopen(): Filename cannot be empty in C:\xampp\htdocs\clinicosight\doctors.php on line 23
Warning: fread() expects parameter 1 to be resource, boolean given in C:\xampp\htdocs\clinicosight\doctors.php on line 24
Warning: fclose() expects parameter 1 to be resource, boolean given in C:\xampp\htdocs\clinicosight\doctors.php on line 26
Warning: mysql_fetch_array() expects parameter 1 to be resource, boolean given in C:\xampp\htdocs\clinicosight\doctors.php on line 46
Notice: Undefined variable: maxpatid in C:\xampp\htdocs\clinicosight\doctors.php on line 50
Warning: mysql_fetch_array() expects parameter 1 to be resource, boolean given in C:\xampp\htdocs\clinicosight\doctors.php on line 51
please edit the codes below and help me with it.Thanks
<?php
include(“validation/header.php”);
include(“dbconnection.php”);
$result = mysql_query(“SELECT MAX(docid) FROM doctor”);
while($row = mysql_fetch_array($result))
{
$maxpatid = $row[0];
$maxpatid++;
}
//insert doctors record
if(isset($_POST[“button”]))
{
// UPLOAD IMAGE CODE
// Temporary file name stored on the server
$tmpName = $_FILES[‘image’][‘tmp_name’];
// Read the file
$fp = fopen($tmpName, ‘r’);
$data = fread($fp, filesize($tmpName));
$data = addslashes($data);
fclose($fp);
//UPLOAD IMAGE CODE ENDS HERE AND IMAGE BINARY VALUE STORES IN VARIABLE $data
$sql=”INSERT INTO doctor(doctorname, quali, specialistin, contactno, emailid, image, biodata,password )
VALUES
(‘$_POST[dfn] $_POST[dmn] $_POST[dln]’ ,’$_POST[quali]’,’$_POST[spin]’,’$_POST[contact]’,’$_POST[emailid]’,’$data’,’$_POST[bio]’,’$_POST[password]’)”;
if (!mysql_query($sql,$con))
{
die(‘Error: ‘ . mysql_error());
}
}
?>
<?php
if(isset($_POST[“button”]))
{
$result = mysql_query(“SELECT MAX(docid) FROM doctor”);
while($row = mysql_fetch_array($result))
{
$maxpatid = $row[0];
}
$docrec = mysql_query(“SELECT * FROM doctor where docid =’$maxpatid'”);
while($row = mysql_fetch_array($docrec))
{
echo “<form id=’formID1′ class=’formular’ method=’post’ enctype=’multipart/form-data’>”;
echo “<b>Doctor Record inserted successfully.. <br><br>”;
//image code ends here
echo “Doctor ID is : $row[docid]<br><br>”;
echo “Doctor Name : $row[doctorname]<br><br>”;
echo “Qualification : $row[quali]<br><br>”;
echo “Specialist in : $row[specialistin]<br><br>”;
echo “Contact No : $row[contactno]<br><br>”;
echo “Email ID : $row[emailid]<br><br>”;
echo “Biodata : $row[biodata]<br><br>”;
echo “</b></form>”;
}
}
else
{
?>
<form id=”formID” class=”formular” method=”post” enctype=”multipart/form-data”>
<div align=”center”><strong>Doctors</strong></div>
<p>Doctor ID
<label for=”dfn2″></label>
<input type=”text” name=”dfn2″ id=”dfn2″ class=”validate[required] text-input” readonly value=”<?php echo $maxpatid; ?>”/>
<label for=”textfield4″>Password</label>
<input type=”password” name=”password” id=”password” class=”validate[required] text-input”/>
<label for=”textfield3″> </label>
Confirm Password
<input type=”password” name=”textfield5″ id=”textfield5″ class=”validate[required,equals[password]] text-input” />
First Name
<label for=”dfn”></label>
<input type=”text” name=”dfn” id=”dfn” class=”validate[required] text-input”>
Middle Name
<input type=”text” name=”dmn” id=”dmn” class=”validate[required] text-input”>
Last Name
<input type=”text” name=”dln” id=”dln” class=”validate[required] text-input”>
Qualification
<input type=”text” name=”quali” id=”quali” class=”validate[required] text-input”>
Specialist in
<input type=”text” name=”spin” id=”spin” class=”validate[required] text-input”/>
<label for=”spid”></label>
Contact No
<input type=”text” name=”contact” id=”contact” class=”validate[required] text-input”>
Email ID
<input type=”text” name=”emailid” id=”emailid” class=”validate[required,custom[email]] text-input”>
Image
<label for=”image”></label>
<input name=”image” accept=”image/jpeg” type=”file”>
Bio-data
<label for=”bio”></label>
<textarea name=”bio” id=”bio” cols=”45″ rows=”5″ ></textarea>
</p>
<p> </p>
<div align=”center”>
<input type=”submit” name=”button” id=”button” value=”Add” class=”submit”>
<input type=”submit” name=”button2″ id=”button2″ value=”Cancel” class=”submit”>
</div><br /><br />
</form>
<?php
}
?>
Hello,
Unfortunately we are not able to provide custom code support. You may want to check the php documentation online to see what you need to change in order for your form to work properly.
Since you are going to work on it anyway, you may want to explore using mysqli instead of mysql as the one mysql will be replaced eventually.
Kindest Regards,
Scott M
there is no error in my code ,,,and still the data is not inserted into the database
Hello Maxeekhan,
Have you tried doing a var_dump($query); right after the insert variable to make sure its spitting out all of the required information?
Best Regards,
TJ Edens
I need a help. I need the php codes for the patients to book an appointment with the specific doctors after they have been registers into the system. After,the patient booked an appointment,the selected doctor should able to view the appointment and able to approve or reject the appointment by click the approve and reject button. Im also need the validation codes for dates to check whether the dates are available or not.Can someone help me,please?
Hello Dineas,
Thank you for contacting us. We are happy to help guide you, but you will need an actual developer to code a custom solution for this.
This is because it will require more access and troubleshooting before the code can be created.
Thank you,
John-Paul
Why won’t my form data insert properly into my MySQL database? .
Hello olivia,
Thank you for contacting us. We will need some additional information. What steps are you taking? Are you following the above guide?
Are you getting an error message?
Please include any additional information to help you troubleshoot further.
Thank you,
John-Paul
Hello, thanks for the codes but i’m encoutering some problems. First, i am not sure my database even connects to the form page. Secondly, if its connecting, data is not been inserted into the data base. I can’t seen to find the problem, please help me.
Here is the html for the form:
<html>
<head>
<title>test</title>
</head>
<body>
<h1>This is page1.php</h1>
<div><a href=’page2.php?id=2′>Click here</a> to go to page2.php</div>
<div style=’margin:20px; width:100px; height:100px; background:blue;’></div>
<form action=’contact.php’ method=’post’>
NAME: <input type=’text’ name=’name’ id=’name’ required /><br />
Email: <input type=’email’ name=’email’ id=’email’ required /><br />
Website: <input type=’text’ name=’website’ id=’website’ required /><br />
Comment:<br />
<textarea name=’comment’ id=’comment’ required ></textarea><br />
<input type=’submit’ value=’Submit’ />
</form>
</body>
</html>
And here is the PHP (contact.php):
<?php
$users_name = $_POST[‘name’];
$users_email = $_POST[’email’];
$users_website = $_POST[‘website’];
$users_comment = $_POST[‘comment’];
$database= “comments”;
$password= “password”;
$username= “username”;
if($users_name&&$users_email&&$users_website&&$users_comment){
$con = mysql_connect(“localhost”, $username, $password) or die(“Could not connect”);
@mysql_select_db(“comments”, $con) or die(“Unable to Connect”);
mysql_query(“INSERT INTO `comments`.`test` (`id`, `Name`, `Email`, `Website`, `Comment`)
VALUES (“”, ‘$users_name’, ‘$users_email’, ‘$users_website’, ‘$users_comment’);”) or die(“Strange error”);
echo “Comment Posted”;
mysql_close($con);
header(“location:index.html”);
} else{
echo “You need to fill all options”;
}
?>
Please, is there a way I can test if my database is connected?
Anticipating your quick response.
Hello faisal,
Thank you for contacting us. We can help you troubleshoot, but will need some additional information. What happens when you try to use the script? Do you get any errors?
Also, have you verified the database settings are correct? Specifically these:
$username = “root”;
$password = “”;
$dbname = “University”;
Thank you,
John-Paul
Hello Lord,
Thank you for contacting us. I looked at your code, and if the database connection fails, you should see a message stating: “Could not connect”
If you do not see this error message, you most likely are connecting successfully.
Thank you ,
John-Paul
it is not working . plz es ko thek kr mujy send kre
<html>
<head>
<title> Table of Student</title>
</head>
<body>
<table border=”1″>
<tr>
<th>Std_ID</th>
<th>Std_Name</th>
<th>Std_Address</th>
<th>Std_Email</th>
</tr>
</table>
</body>
</html>
<?php
$servername = “localhost”;
$username = “root”;
$password = “”;
$dbname = “University”;
// Create connection
$conn = mysqli_connect($servername, $username, $password, $dbname);
// Check connection
if (!$conn) {
die(“Connection failed: ” . mysqli_connect_error());
}
$sql = “INSERT INTO student*
VALUES (‘1’, ‘Faisal’, ‘M.B.DIN’,’[email protected]’)”;
$conn->close();
?>
Could someone please tell me what other reason besides wrong credentials would cause me to get an access denied error message with this code? I have checked the users access and set the password several times to ensure that it is right.
Could not connect: Access denied for user ‘db_username’@’localhost’ (using password: YES)
[code]
<?
if( $_POST )
{
$con = mysql_connect("localhost","db_username","lamepassword");
if (!$con)
{
die('Could not connect: ' . mysql_error());
}
mysql_select_db("database_name", $con);
$users_name = $_POST['name'];
$users_email = $_POST['email'];
$users_website = $_POST['website'];
$users_comment = $_POST['comment'];
$users_name = mysql_real_escape_string($users_name);
$users_email = mysql_real_escape_string($users_email);
$users_website = mysql_real_escape_string($users_website);
$users_comment = mysql_real_escape_string($users_comment);
$articleid = $_GET['id'];
if( ! is_numeric($articleid) )
die('invalid article id');
$query = "
INSERT INTO `database_name`.`comments` (`id`, `name`, `email`, `website`,
`comment`, `timestamp`, `articleid`) VALUES (NULL, '$users_name',
'$users_email', '$users_website', '$users_comment',
CURRENT_TIMESTAMP, '$articleid');";
mysql_query($query);
echo "<h2>Thank you for your Comments!</h2>";
mysql_close($con);
}
?>
[/code]
Jack,
To be honest, if that error appears, then the problem has to do with the credentials. I know you’re asking for another possible reason, but in this case it typically no other reason than credentials. You may need to double-check what USER is assigned to run the script as well. I hope this helps to answer your question, please let us know if you require any further assistance.
Regards,
Arnel C.
please help me solve this: uploading a file is the problem.
<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.0 Transitional//EN” “https://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd”>
<html xmlns=”https://www.w3.org/1999/xhtml”>
<head>
<meta http-equiv=”Content-Type” content=”text/html; charset=utf-8″ />
<title>HOME</title>
<link rel=”stylesheet” href=”../CSS/general.css” />
</head>
<body>
<?php
include(“../menu.php”);
?>
<div id = “content”>
<div id=”left-side”>
<h2>farming tools</h2>
<div id=”upload”>
<table >
<form enctype=”multipart/form-data” action=”” method=”POST” name=”f tools”>
<legend></legend>
<tr align=”center”><td> <label>FIRST NAME:</label></td><td>
<input name=”name” type=”text” />
</td></tr><tr align=”center”><td>
<label>ITEM NAME:</label></td><td>
<input name=”item” type=”text” />
</td></tr><tr align=”center”>
<td>
<label>Price:</label></td>
<td><input name=”PRICE” type=”text” required=”required”/>
</td>
</tr><tr align=”center”>
<td><label>LOCATION:</label></td>
<td><input name=”location” type=”text” /> </td></tr>
<tr align=”center”>
<td><label>PHONE NO:</label></td>
<td><input name=”phone” type=”text” /> </td></tr>
<tr align=”center”>
<td><label>EMAIL:</label></td>
<td><input name=”email” type=”email” required=”required”/> </td>
</tr>
<tr>
<td>Select image to upload:</td><td>
<input type=”file” name=”upload” />
</td></tr><tr align=”center”><td>
<label>details:</label></td><td>
<textarea id = “Message” name = “Message” type = “text” placeholder = “Type Message Here” data-validate = “required”>
</textarea>
</td>
</tr> <tr><td>
<input class=”submit” type=”submit” value=”update” name=”update”/>
</form>
<?php
if(isset($_POST[“update”])){
if(!empty($_POST[’email’]) && !empty($_POST[‘PRICE’]) ) {
$Name=$_POST[“name”];
$item=$_POST[“item”];
$email=$_POST[’email’];
$price=$_POST[‘PRICE’];
$location=$_POST[‘location’];
$phone=$_POST[‘phone’];
$upload=$_FILES[‘upload’][‘name’];
$tmp_name = $_FILES[‘upload’][‘tmp_name’];
$message=$_POST[‘Message’];
include(“../connect.php”);
move_uploaded_file($_FILES[‘upload’][‘tmp_name’],”../upload/”.$_FILES[‘upload’][‘name’]) or die(mysql_error());
$sql=”INSERT INTO tool (Name, itemName, PRICE, location, phone, email, image, description)
VALUES (‘$Name’,’$item’,’$price’,’$location’,’$phone’,’$email’,’$upload’,’$message’)”;
if($result=mysqli_query($con,$sql)){
echo “Thank you, your item was posted successfully”;
} else {
echo “Failure!”;
}
}
mysqli_close($con);
}
?>
</td>
</tr> </table>
<?php
echo “<BR/>”;
?>
<!– what to buy –>
</div>
</div>
<div align=”right”>
<img src=”../slide/img/home/tanks.png” width=”501″ height=”450″/>
</div>
</div>
<?php
include(“../footer.php”);
?>
</body>
</html>
Hello Shwe,
What error are you getting when you try to upload files? Are you trying to upload files into the database or just onto the server?
Best Regards,
TJ Edens
I need help.I cant able to find errors in this codes.It failed to insert data into the database created in phpmyadmin and im using xampp.
<html>
<body>
<?php
$con = mysql_connect(“localhost”,”root”,””);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“patients”, $con);
$sql=”INSERT INTO `patients`(`id`, `patient_name`, `patient_pass`, `patient_email`) VALUES ([value-1],[value-2],[value-3],[value-4])
(‘$_POST[id]’,’$_POST[patient_name]’,’$_POST[patient_pass]’,’$_POST[patient_email]’)”;
if (!mysql_query($sql,$con))
{
die(‘Error: ‘ . mysql_error());
}
else
{
echo “You are successfully registered!!!”;
}
mysql_close($con);
?>
</font>
</br>
</br>
<input type=”button”value=”Finish”onclick=”history.go(-2);return true;”>
</table>
</body>
</html>
Hello dineas,
You will first want to rule out the query itself by testing it alone within the phpmyadmin. You then will also want to test the error code when it does the Insert in the php.
Kindest Regards,
Scott M
Dear Sir / Madam,
I have followed exactly the steps but when I enter the details, it shows
“Notice: Undefined index: id in C:\xampp\htdocs\manage_comments.php on line 23
invalid article id“
Line 23: $articleid = $_GET[‘id’];
I have tried many ways, like inserting “issets( )” but it still doesn’t work.
May I kindly request for your help please? Thank you?
Hello Danzo,
Thank you for contacting us. Since this is just one guide in a 7 section series, have you completed the previous guides too?
Unfortunately, it is difficult for us to troubleshoot code, especially in a xampp environment, since I have no way of knowing if it was setup correctly. Similar to other questions above, you will want to use normal code troubleshooting techniques such as printing out the query variable to see what is being created. Also, you can add error trapping to see if the query function is throwing any error codes.
Thank you,
John-Paul
Hi, Not sure if you could help. I have followed the guide but when I click submit I get half an error of:
Thank you for your Comment!”; mysql_close($con); } ?>
Which looks like its not understanding the close connection as a function and just echoing it.
Also it is not uploading to my SQL database.
My code as follows:
###########
<?php
if( $_POST )
{
$con = mysql_connect(“localhost”,”root”,”password”);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“paranormal”, $con);
$users_name = $_POST[‘name’];
$users_email = $_POST[’email’];
$users_region = $_POST[‘dropdown’];
$users_where = $_POST[‘where’];
$users_when = $_POST[‘date’];
$users_comment = $_POST[‘comment’];
$users_name = mysql_real_escape_string($users_name);
$users_email = mysql_real_escape_string($users_email);
$users_region = mysql_real_escape_string($users_region);
$users_where = mysql_real_escape_string( $users_where);
$users_when = mysql_real_escape_string($users_when);
$users_comment = mysql_real_escape_string($users_comment);
$articleid = $_GET[‘id’];
if( ! is_numeric($articleid) )
die(‘invalid article id’);
$query = “INSERT INTO `paranormal`.`comments` (`id`, `name`, `email`, `when`, `where`, `comment`, `timestamp`, `articleid`, `Region`) VALUES (NULL, ‘$users_name’, ‘$users_email’, ‘$users_when’, ‘$users_where’, ‘$users_comment’, CURRENT_TIMESTAMP, ‘$articleid’, ‘$users_region’);”;
mysql_query($query);
echo “<h2>Thank you for your Comment!</h2>”;
mysql_close($con);
}
?>
Hello Eggface,
It doesn’t appear that you included ANY database connection information. The message you’re seeing is PART of the code. If you’re trying to do something different, then you would need to modify the code for your purpose. You can remove the echo, as necessary. It was added to the example code above. The example code requires that you add your database connection information in order to properly function.
Regards,
Arnel C.
This is the error message I receive upon submit:
Warning: mysql_connect(): Access denied for user ‘afx_u1’@’localhost’ (using password: YES) in /home/afx/public_html/dev/manage_comment.php on line 4
Could not connect: Access denied for user ‘afx_u1’@’localhost’ (using password: YES)
My password is not YES, and it is entered correctly (i’m positive)
What can I test or change to make this work?
Hello Tubby,
Thank you for contacting us. Make sure you have created the user and gave them permission to access the database.
Also, check the database, and database username for typos or misspellings.
Thank you,
John-Paul
<?php
include(‘connection.php’);
mysql_select_db(’employee’);
error_reporting(1);
$query=”INSERT INTO emp_info VALUES (”, ‘$name’,’$Address’,’$email’,’$mobial’)”;
mysql_query($query);
?>
<html>
<body>
<form method=”post” enctype=”multipart/form-data”>
<table align=”left”>
<tr align=”left”>
<th>Name :</th>
<th><input type=”text” name=”name” required></th>
</tr>
<tr align=”left” valign=”top”>
<th>Address :</th>
<th><textarea rows=”4″ cols=”21″ name=”Address” required></textarea></th>
</tr>
<tr align=”left”>
<th>email :</th>
<th> <input type=”text” name=”email” required> </th>
</tr>
<tr align=”left”>
<th width=”100″>Mobial :</th>
<th width=”100″> <input type=”text” pattern=”[0-9]*” maxlength=”10″ name=”mobial” required> </th>
</tr>
<tr>
<th colspan=”2″><input type=”submit” value=”INSERT”></th>
</tr>
</table>
</form>
</body>
</html>
plese help me to insert data to database with php
i am a many try but program sucssess but data not inserted
Hello,
You will need to have rthe query print to the screen to see if it has the information you expect. Also, it is a good idea to error trap the query call so you can see any error messages that the function throws.
Kindest Regards,
Scott M
Hello manoj,
You will want to use normal code troubleshooting techniques such as printing out the query variable to see what is being created. Also, you can add error trapping to see if the query function is throwing any error codes.
Kindest Regards,
Scott M
Hi, the pages now work and when I click submit the “Thank You” message appears but nothing write to my database?
My manage_comments.php is as follows:
<body>
<?php
if( $_POST )
{
$con = mysqli_connect(“localhost”,”root”,””);
mysqli_select_db($con, “verifytrade”);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
$users_name = $_POST[‘name’];
$users_email = $_POST[’email’];
$users_comment = $_POST[‘comment’];
$users_name = mysql_real_escape_string($users_name);
$users_email = mysql_real_escape_string($users_email);
$users_comment = mysql_real_escape_string($users_comment);
$query = “
INSERT INTO `verifytrade`.`comments` (`id`, `name`, `email`,`comment`,
`timestamp`) VALUES (NULL, ‘$users_name’,
‘$users_email’, ‘$users_comment’,
CURRENT_TIMESTAMP);”;
mysql_query($query);
echo “<h2>Thank you for your Comment!</h2>”;
mysqli_close($con);
}
?>
</body>
Thanks in advance. This has been a lot of help!
Hi Arnel,
My manage_comments code is as follows:
<?
if( $_POST )
{
$con = mysql_connect(“localhost”,””,””);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“verifytrade”, $con);
$users_name = $_POST[‘name’];
$users_email = $_POST[’email’];
$users_website = $_POST[‘website’];
$users_comment = $_POST[‘comment’];
$users_name = mysql_real_escape_string($users_name);
$users_email = mysql_real_escape_string($users_email);
$users_website = mysql_real_escape_string($users_website);
$users_comment = mysql_real_escape_string($users_comment);
$articleid = $_GET[‘id’];
if( ! is_numeric($articleid) )
die(‘invalid article id’);
$query = “
INSERT INTO `verifytrade`.`comments` (`id`, `name`, `email`, `website`,
`comment`, `timestamp`, `articleid`) VALUES (NULL, ‘$users_name’,
‘$users_email’, ‘$users_website’, ‘$users_comment’,
CURRENT_TIMESTAMP, ‘$articleid’);”;
mysql_query($query);
echo “<h2>Thank you for your Comment!</h2>”;
mysql_close($con);
}
?>
Thanks!
Hello,
Make sure to put the “<?php” in place the current statement and then your code should work. If you have any further questions or comments, please let us know.
Regards, Arnel C.
Hi,
I have followed the steps and somehow “Thank you for your Comment!”; mysql_close($con);}?>” is displaying at the top of each page before I even submit anything. I can’t seem to figure out why. Any feedback would greatly appreciated.
Thanks in advance!
Hello Eugene,
If you are seeing that portion of the code from above, then it’s most likely happening because you didn’t close the quotes with the echo command. Double-check your code and let us know if you have any further questions or comments.
Regards,
Arnel C.
Very Nice
the site is ok.good work
Helo
How can I place a “thank you page” in this PHP?
What would a example be ?
Hello Joe,
Thank you for contacting us. We are happy to help, but will need some additional information.
What type of “thank you page” do you want to place, and where?
Can you provide a link to an example page, etc.
Thank you,
John-Paul
Hello, When I log in my cpanel there is no option for “comments”
Hello Stephen,
Thanks for the question. We are a little puzzled by it. We’re not sure if you’re looking for a place to leave feedback, or if you are thinking that the interface is similar to WordPress where you can manage comments. The cPanel is the interface for installing software, accessing your hosting account files, managing email, ftp, security, domain options, security, and many other options. It’s basically the control center for your hosting account. If you’re looking at managing a website, then the interface depends on the software you used to build the website. If you can provide more information about what you are looking for specifically, then we would be happy to provide whatever support we can.
Kindest regards,
Arnel C.
Hi
I am creating login and register page. when I am trying to enter data into register.php then data is not inserted into table as well as email not send.
Find a solution.
register.php
<?php
$conn =new mysqli(‘localhost’,’admin_password’,’test123′,’password_app’);
include(“../PHPMailer/PHPMailerAutoload.php”);
//require_once’connection.php’;
//check for spam
//if(empty($_POST[‘spamProtection’]))
//{
if(isset($_POST[‘Register’]))
{
$count=0;
//check if required fields are not empty
if(trim($_POST[‘name’]) == ”) {
$hasError = true;
} else {
$name = trim($_POST[‘name’]);
}
if(trim($_POST[‘user’]) == ”) {
$hasError = true;
} else {
$user=trim($_POST[‘user’]);
}
if(trim($_POST[’email’]) == ”) {
$hasError = true;
} else {
$email = trim($_POST[’email’]);
}
if(trim($_POST[‘password’]) == ”) {
$hasError = true;
} else {
$email = trim($_POST[‘password’]);
}
$level=trim($_POST[‘level’]);
//If there is no error, open the connection and send the email
if(!isset($hasError)) {
$sql =”INSERT INTO users (name,user,pass,email,level) VALUES (‘$name’,’$user’,’$pass’,’$email’,’$level’)”;
echo $conn;
echo $name,$user,$pass,$level;
if (!mysqli_query($conn,$sql))
{
?>
<script>
alert(“Account already exist!”);
window.location.href=”home.php”;
</script>
<?php
}
else{
$mail = new PHPMailer;
$mail->isSMTP(); // Set mailer to use SMTP
$mail->Host = ‘mail.the-right-solution.com’; // Specify main and backup SMTP servers
$mail->SMTPAuth = true; // Enable SMTP authentication
$mail->Username = ‘[email protected]’; // SMTP username
$mail->Password = ‘Pwd@12345’; // SMTP password
$mail->Port = 25; // TCP port to connect to
$mail->From = ‘[email protected]’;
$mail->addAddress($email); // Add a recipient
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = ‘User Information’;
$mail->Body = ‘<h1>Hello </h1> <p> Name : ‘ .$name .
‘</p> <p> User : ‘ .$user .
‘</p> <p> Password : ‘ .$pass .
‘</p> <p> Level : ‘ .$level ;
if(!$mail->send()) {
$message.= ‘Message could not be sent. ‘;
$message.= ‘Mailer Error: ‘ . $mail->ErrorInfo;
} else {
$message.= ‘Message has been sent. ‘;
}
?>
<script>
alert(“To complete your registration, please check your mailbox for further instructions!”);
window.location.href=”home.php”;
</script>
<?php
mysqli_close($conn);
// $mysql->close;
}
}
else{
?>
<script>
alert(‘stuck here’);
/*window.location.href=”home.php”;*/
</script>
<?php
}
}
else
{
header(‘Location: home.php’);
}
?>
…login.php…
<?php
@session_start();
include_once ‘connection.php’;
// Create connection
$mysqli = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($mysqli->connect_error) {
die(“Connection failed: ” . $mysqli->connect_error);
}
$allowed_codes=$mysqli->query(“select * from users”);
?>
<!DOCTYPE html>
<html>
<meta charset=”UTF-8″>
<head>
<title>User Information</title>
<style>
.error {color: #FF0000;}
</style>
<meta name=”viewport” content=”width=device-width, initial-scale=1″>
<link rel=”stylesheet” href=”https://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap.min.css”>
<script type=”text/javascript” src=”https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js”></script>
<script type=”text/javascript” src=”https://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/js/bootstrap.min.js”></script>
<meta http-equiv=”Content-Type” content=”text/html; charset=iso-8859-1″>
<link href=”../css/main.css” rel=”stylesheet” type=”text/css”>
<script src=”../js/jquery.validate.js”></script>
<script src=”../js/home.js”></script>
</head>
<style>
/*?>input[type=”text”]{
width:93%;
}
input[type=”password”]{
width:93%;
} */
#register{
display:none;
}
.error{
color:#F00;
}
</style>
</head>
<body id=”main”>
<h1> User Information </h1>
<section id=”login”>
<form id=”authform” form method=”post” action=”Authentication.php” >
<!–<p> please enter your email and password below.</p>–>
<div class=”row”>
<div class=”sm-8″>
<label>Email</label>
<input type=”text” name=”email” required=”required” class=”input-large” />
</div>
</div>
<div class=”row”>
<div class=”sm-8″>
<label> </label>
<input name = “client” width=”140″ type=”radio” id=”client_login” value=”old” checked=”checked” />I have a Password
</div>
</div>
<div class=”row”>
<div class=”sm-8″>
<label> </label>
<input name = “client” width=”140″ type=”radio” value=”new” />I’m New
</div>
</div>
<div class=”row”>
<div class=”sm-4″>
<label>Password</label>
<input id= “password” input name=”password” type=”password” size=”8″ maxlength=”8″ class=”form-control” required=”required”/>
</div>
</div>
<div class=”row”>
<div class=”sm-4″>
<a href=”” onclick=”winOpen(‘forgotPassword.php’,’auto’,500,300)” > <span style=”color:#D00;font-size:10px” >Forgot Your Password?</span> </a>
</div>
</div>
<div class=”row”>
<div class=”sm-8″>
<input name=”Login” type=”submit” value=”Login” class=”btn_login” align=”right” colspan=”2″/>
</div>
</div>
</form>
</section><!–#login–>
<section id=”register”>
<form method=”post” action=”register.php” name=”registerform” id=”registerform” onsubmit1=”return validateForm()” onsubmit=”return CheckPassword()”/>
<div class=”row”>
<div class=”sm-8″>
<label>Email</label>
<input name = “email1″ required=”required” type=”text” size=”40″ class=”input-large”/>
</div>
</div>
<div class=”row”>
<div class=”sm-8″>
<label> </label>
<input name = “client” width=”140″ type=”radio” value=”old” />I have a Password
</div>
</div>
<div class=”row”>
<div class=”sm-8″>
<label> </label>
<input name = “client” width=”140″ type=”radio” id=”client_register” value=”new” checked=”checked”/>I’m New
</div>
</div>
<div class=”row”>
<div class=”sm-4″>
<label>Name</label>
<input name=”name” type=”text” size=”20″ maxlength=”20″ class=”form-control” required=”required” />
</div>
</div>
<div class=”row”>
<div class=”sm-4″>
<label>User</label>
<input name=”user” type=”text” size=”20″ minlength=”8″ maxlength=”20″ class=”form-control” required=”required”/>
</div>
</div>
<div class=”row”>
<div class=”sm-4″>
<label>Password</label>
<input id= “password” input name=”password” type=”password” size=”8″ maxlength=”8″ class=”form-control” required=”required”/>
</div>
</div>
<div class=”row”>
<div class=”sm-4″>
<label>Level</label>
<select name=”level” required=”required” id=”level” class=”form-control scroll” />
<option value=”0″>1</option>
</select>
</div>
</div>
<div class=”row”>
<div class=”sm-8″>
<input name=”Register” type=”submit” value=”REGISTER” class=”button btn-danger” align=”right” colspan=”2″/>
<ul> </ul>
</div>
</div>
<br /><br />
</form>
</section>
</div>
<?php $mysqli->close(); ?>
</body>
<script type=”text/javascript”>
function validateForm() {
var x = document.forms[“registerform”][“name”].value;
if (x==null || x==””) {
alert(“Name must be filled out”);
return false;
}
var x = document.forms[“registerform”][“user”].value;
if (x==null || x==””) {
alert(“User must be filled out”);
return false;
}
var x = document.forms[“registerform”][“password”].value;
if (x==null || x==””) {
alert(“Password must be filled out”);
return false;
}
var x = document.forms[“registerform”][“level”].value;
if (x==null || x==””) {
alert(“Level must be filled out”);
return false;
}
var x = document.forms[“registerform”][“email”].value;
var atpos = x.indexOf(“@”);
var dotpos = x.lastIndexOf(“.”);
if (atpos< 1 || dotpos<atpos+2 || dotpos+2>=x.length) {
alert(“Not a valid e-mail address”);
return false;
}
}
</script>
<script type=”text/javascript”>
function CheckPassword() {
var error = “”;
var illegalChars = /[\W_]/; // allow only letters and numbers
if (document.forms[“registerform”][“password”].value == “”) {
document.forms[“registerform”][“password”].style.background = ‘Yellow’;
error = “You didn’t enter a password.\n”;
alert(error);
return false;
} else if ((document.forms[“registerform”][“password”].value.length < 8) ) {
error = “The password is the wrong length. \n”;
document.forms[“userForm”][“password”].style.background = ‘Yellow’;
alert(error);
return false;
} else if (illegalChars.test(document.forms[“registerform”][“password”].value)) {
error = “The password contains illegal characters.\n”;
document.forms[“registerform”][“password”].style.background = ‘Yellow’;
alert(error);
return false;
} else if ( (document.forms[“registerform”][“password”].value.search(/[a-zA-Z]+/)==-1) || (document.forms[“registerform”][“password”].value.search(/[0-9]+/)==-1) ) {
error = “The password must contain at least one numeral.\n”;
document.forms[“registerform”][“password”].style.background = ‘Yellow’;
alert(error);
return false;
} else {
document.forms[“registerform”][“password”].style.background = ‘White’;
}
return true;
}
</script>
</html>
Are you receiving any error messages?
hey ive got a problem and its eating away my head! when i load page1 it displays the form but also this code: echo “<h2>Thank you for your Comment!</h2>”; ?>
idont know whats wrong? also i think due to this queer display i cant submit anything
this is my manage_comments.php
<?
if( $_POST )
{
$con = mysql_connect(“localhost”,”x”,”y”);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“search”, $con);
$users_name = $_POST[‘name’];
$users_email = $_POST[’email’];
$users_website = $_POST[‘website’];
$users_comment = $_POST[‘comment’];
$users_name = mysql_real_escape_string($users_name);
$users_email = mysql_real_escape_string($users_email);
$users_website = mysql_real_escape_string($users_website);
$users_comment = mysql_real_escape_string($users_comment);
$articleid = $_GET[‘id’];
if( ! is_numeric($articleid) )
{
die(‘invalid article id’);
}
$query = “
INSERT INTO `search`.`comments` (`id`, `name`, `email`, `website`,
`comment`, `timestamp`, `articleid`) VALUES (NULL, ‘$users_name’,
‘$users_email’, ‘$users_website’, ‘$users_comment’,
CURRENT_TIMESTAMP, ‘$articleid’);”;
mysql_query($query);
echo “<h2>Thank you for your Comment!</h2>”;
mysql_close($con);
}
?>
Hello David,
Sorry for the problems with the code. We unfortunately can’t really troubleshoot your code. However, if your code is not working, it’s most likely centered around the conditions of the if statements in the beginning of the code. If those conditions aren’t being met, then your form won’t post. Apologies again that we can’t give you a direct answer. If you have any further questions, please let us know.
Regards,
Arnel C.
Oh ok, well thank you so much again!!
Oh! Perfect, thanks so much for all of your help! 🙂 Can I rate you on this site or something?
There aren’t any user ratings or anything, but I’m glad I was able to answer your questions!
Oh ok! I’m so sorry if this is a dumb question, but how do I change the URL for the first page? Or if it is too involved to explain, can you point me to a good tutorial if you know any?
When a user is leaving a comment, you would simply point them to https://annaburnham.com/phpForm/page1.php?id=1
Oh yup I see that! I thought I did that correctly.. That would be this code correct?
<? include(“manage_comments.php”); ?>
<h1>This is page1.php</h1>
<div><a href=’page2.php?id=1′>Click here</a> to go to page2.php</div>
<div style=’margin:20px; width:100px; height:100px; background:blue;’></div>
<? include(“formcode.php”); ?>
and this:
<? include(“manage_comments.php”); ?>
<h1>This is page2.php</h1>
<div><a href=’page1.php?id=1′>Click here</a> to go to page1.php</div>
<div style=’margin:20px; width:100px; height:100px; background:orange;’></div>
<? include(“formcode.php”); ?>
Yes, that is fine.
As for the URL that I am referring to in the above comment, I am referring to the URL in the address bar. When a user commends on your site, they should be visiting something like page1.php?id=1 so that the code knows what page the comment is being left on.
https://annaburnham.com/phpForm/
I just found out that it works if you go to page 2 first (and type stuff in and submit), and then go back to page 1. But if you start typing and submit info on page 1 first, the “invalid article id” error happens. At least it works for the most part?
This is because of the URL. The code that inserts data into the database requires that an ID be defined within the URL. If you take a look at the URL after you click on Page 2, you will notice that it says id=”1″. Without this defined, it does not know the article ID that the comment would be left on.
Wait, now the comments are showing up! I have no idea why it didn’t work at first after I changed it to the code you provided. Thank you! 🙂
Still the same error “invalid article id” when I type in information and click submit. But actually I just tried doing a test comment again in my cPanel and I got this error:
1 row inserted.
Inserted row id: 2
Warning: #1366 Incorrect integer value: ” for column ‘articleid’ at row 1
It is in red instead of green like in your tutorial on adding a test comment.
It sounds like you are not passing the correct information from the GET variable. Could you provide me with the full URL on this page you are referring to?
That code still doesn’t work :/ There must be an error somewhere else then… Thanks for the advice!!
Could you provide me with the exact error you are getting now?
Hey! I have read through everyone else’s comments/error messages and I still have not been able to figure out what is wrong with my code. I am following this tutorial for a class, and I did everything in order but I am getting “invalid article id”, and it is also not inserting any information into the database. Is there something I am missing? Here is my code:
<?
if( $_POST )
{
$con = mysql_connect(“localhost”,”aburnham_user”,”mypassword”);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“aburnham_form”, $con);
$users_name = $_POST[‘name’];
$users_email = $_POST[’email’];
$users_website = $_POST[‘website’];
$users_comment = $_POST[‘comment’];
$users_name = mysql_real_escape_string($users_name);
$users_email = mysql_real_escape_string($users_email);
$users_website = mysql_real_escape_string($users_website);
$users_comment = mysql_real_escape_string($users_comment);
$articleid = $_GET[‘id’];
if( ! is_numeric($articleid) )
die(‘invalid article id’);
$query = “
INSERT INTO `aburnham_form`.`comments` (`id`, `name`, `email`, `website`,
`comment`, `timestamp`, `articleid`) VALUES (NULL, ‘$users_name’,
‘$users_email’, ‘$users_website’, ‘$users_comment’,
CURRENT_TIMESTAMP, ‘$articleid’);”;
mysql_query($query);
$return = mysql_query($query);
echo “<h2>Thank you for your Comment!</h2>”;
mysql_close($con);
}
?>
The reason your are getting this error is because of this code:
$articleid = $_GET[‘id’];
if( ! is_numeric($articleid) )
die(‘invalid article id’);
It should look something like this:
$articleid = $_GET[‘id’];
if( ! is_numeric($articleid) ) {
die(‘invalid article id’); }
Hello Sir,
I have a querry regarding insert when i run my registration code that my entries are added in two different tables that are registration and individual table at a time which should not happen actually values are for registration table and in tha individual table some filled and some blank are there and i am not getting the problem why that happen so can you please help me ?
Hello Gauri,
Thank you for contacting us. We are happy to help, but will need some additional details to troubleshoot the problem.
Can you explain what you are trying to accomplish?
Can you provide the query you are executing?
Can you explain the steps you are taking? This will allow us to follow along with you.
Thank you,
John-Paul
Not getting errors, its simply not inserting to database?
<?
if( $_POST )
{
$con = mysql_connect(“localhost”,”walding_test”,”test123″);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“walding_Master”, $con);
$users_name = $_POST[‘name’];
$users_email = $_POST[’email’];
$users_website = $_POST[‘website’];
$users_comment = $_POST[‘comment’];
$users_name = mysql_real_escape_string($users_name);
$users_email = mysql_real_escape_string($users_email);
$users_website = mysql_real_escape_string($users_website);
$users_comment = mysql_real_escape_string($users_comment);
$query = “INSERT INTO `walding_Master`.`Comment` (`ID`, `name`, `email`, `website`, `comment`)
VALUES (NULL, ‘$users_name’,’$users_email’, ‘$users_website’, ‘$users_comment’);”;
mysql_query($query);
echo “<h2>Thank you for your Comment!</h2>”;
echo $query;
mysql_close($con);
}
?>
//////HTML///////
<form action=”contactus.php” method=”POST”>
<div>
<input type=”text” name=”name” id=”name” value=”” placeholder=”Name”>
</div>
<div>
<input type=”email” name=”email” id=”email” value=”” placeholder=”Email”>
</div>
<div>
<input type=”url” name=”website” id=”website” value=”” placeholder=”Website URL”>
</div>
<div>
<textarea rows=”10″ name=”comment” id=”comment” placeholder=”Comment”></textarea>
</div>
<div>
<input type=”submit” name=”submit” value=”Add Comment”>
Hello Marty,
In the code you provided, you are not checking the return code for the mysql_query function. This is why you are not getting a syntactical error message. You will want to set the function to a variable like so:
Then you can echo out the $return variable to see what the error message is. Another thing to check is to echo out the $query string before it runs so you can see what the actual query is.
Kindest Regards,
Scott M
Somebody please help…how to fix
Parse error: syntax error, unexpected ” (T_ENCAPSED_AND_WHITESPACE), expecting ‘]’…
my code is:
$sql=”INSERT INTO information(student name,sex,religious,dateofbirth,email,ic number,address,zipcode,country)
VALUES
(‘$_POST[student name]’,’$_POST[sex]’,’$_POST[religious]’,’$_POST[dateofbirth]’,’$_POST[email]’,’$_POST[ic number]’,’$_POST
[address]’, ‘$_POST[zipcode]’, ‘$_POST[country]’)”;
Hello,
What you have here is a php syntax error. It appears there is a problem with one of your values. It would be much easier if you placed each POST into a specific variable and then placed the variables into the VALUES section. This way, the one POST that is giving the issue would be flagged and you could see which one is incorrect.
Kindest Regards,
Scott M
Thank you John for quick response,
The database name and the table name are identical to the one I see in myphpadmin. I printed the query and it works. I have no idea why it is not inserting into the database. It does not give me any errors but basically it does not insert.
I am working on local host user name is root and has no password
I made sure root at local host has all the privileges
Thank you
If you have confirmed that everything is correct as far as your connection goes, be sure that your variables are getting assigned with the expected data. This can be as simple as echoing out the variables to ensure that the correct data is returned.
Hello, I did exactly whats in the article. I get the message that is successful but it does not insert the data to the database. my code is:
<?
if( $_POST )
{
$con = mysql_connect(“localhost”,”root”,””);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(‘samidb’, $con);
$number = $_POST[‘number’];
$name = $_POST[‘name’];
$desc = $_POST[‘desc’];
$number = mysql_real_escape_string($number);
$name = mysql_real_escape_string($name);
$number = mysql_real_escape_string($desc);
//$articleid = $_GET[‘id’];
//if( ! is_numeric($articleid) )
//die(‘invalid article id’);
$query = “
INSERT INTO ‘samidb’.’sami’ (`number`, `name`, `desc`, `website) VALUES (‘$number’,
‘$name’, ‘$desc’);”;
if (!$query) {
die(‘Could not Insert: ‘ . mysql_error());
}
mysql_query($query);
echo “<h2>Thank you for your Comment!</h2>”;
mysql_close($con);
}
?>
Hello Sami,
Thank you for your question. I recommend checking your Database name, and confirming you are checking the correct database.
Also, you may want to print out the query, to ensure it is running what you expected.
If you have any further questions, feel free to post them below.
Thank you,
John-Paul
Thanks Scott, I found out my mistake and I needed your help again cause I want to do subqueries but I just can’t get it right.
This is my error message for mySQL:
Could not Insert: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ‘SELECT ‘4’ FROM pinStatus WHERE pinNumber=’4” at line 1
This is my new edited code:
*** Extra code removed ***
mysql_query(“UPDATE pinStatus SET pinStatus=’$setting’ WHERE pinNumber=’$pin’;”);
$success=mysql_query(“INSERT INTO sensor VALUES(NULL, ‘1’, ‘Red LED’, NOW(), NULL) SELECT ‘4’ FROM pinStatus WHERE pinNumber=’4′;”);
if (!$success) {
die(‘Could not Insert: ‘ . mysql_error());
}
$success=mysql_query(“INSERT INTO sensor VALUES(NULL, ‘2’, ‘Blue LED’, NOW(), NULL) SELECT ’17’ FROM pinStatus WHERE pinNumber=’17’;”);
if (!$success) {
die(‘Could not Insert: ‘ . mysql_error());
}
*** Extra Code Removed ***
Hi guys, I would like to seek help on my INSERT method to mySQL database. I manage to store data but it always store the 1st data if I click second or third button, it will record all instead of just record that data which I have switch on or off.
*** Code Removed ***
Hello,
When constructing a Select statement, you are typically looking to pull a column name (or * meaning all information from the referenced tables). You are asking it to pull a 4 where the pinNumber =’4′. If you want the number 4 to be pulled you may want to say something like:
This will return the 4 you are looking for.
Kindest Regards,
Scott M
Hello aoiregion,
This sounds like a logic issue and not a syntax issue. Being a logic issue I would need to run the code against a database to figure out where the code is incorrect. That is not something we can do from here.
However, you should have the code echo out (print) the query to the screen so you can see which one it is firing off. Once you know which query it is sending, you can then check the code to see why it is setting those particular conditions. It can take many tries until you iron it out, but that is part of the fun of coding!
Kindest Regards,
Scott M
This was a easy to read clear article. After I read the code with the comments I understood what the process is. Well Done!
Hi guys, I’m very interested in PHP after looking at this tutorial and I decided to a small project for myself. I have created my own Web Control Interface and setup hardware already, by the way, I’m using Raspberry PI as my hardware.
The main issues right now is that I don’t know why my INSERT method won’t works and when I check mySQL database, is empty. Hope you guys could help me out with it as soon as possible, thanks in advance, guys.
This is my control.php:
*** Code Removed ***
Hello,
When working with databases as well as other important functions and tasks, error trapping is important. For instance, you will need to ensure that you are actually connecting to the database without issue. To do so, you need to test the connection return variable. If an error occurs, then you want to know that specific error. You simply need to check that variable as in the sample code below:
if (!$link) {
die(‘Could not connect: ‘ . mysql_error());
}
You can do the same for each INSERT statement you have so if any of them return an error you will see it:
if (!$success) {
die(‘Could not Insert: ‘ . mysql_error());
}
As you can see, they are very similar and simple. Do this for all SQL statements including the connection so you can see any errors you are getting.
One last thing, you may want to look into mysqli instead of mysql when working in php. The mysql functions are older and will be removed in a future version.
Kindest Regards,
Scott M
This code should appropriately insert data into a database. What is the specific error you are getting?
hi scott – thanks for the quick response. i’m pretty newb to this so can you elaborate on what you mean by have my code echo out the query?
THanks!
Hello Amit,
What you want to do is to have a statement like the one below after you set your $query variable:
This should print out the full query statement that is being run against the database. It will show you whether or not the variable fields are being populated before the insert.
Kindest Regards,
Scott M
hi – everything seems to be working, but the rows that get inserted into mysql database are just blank, here is the code i’m using:
<? if( $_POST ) { $con = tpatel”,”denlax11″); if (!$con) { s_firstname); s_lastname); s_email); $users_comments s_comments); $query = ” INSERT INTO
mysql_connect(“localhost”,”ami
die(‘Could not connect: ‘ . mysql_error()); }
mysql_select_db(“Inquiries”, $con); $users_firstname =
$_POST[‘FNAME’]; $users_lastname = $_POST[‘LNAME’]; $users_email =
$_POST[‘EMAIL’]; $users_comments = $_POST[‘COMMENTS’];
$users_firstname = mysql_real_escape_string($user
$users_lastname = mysql_real_escape_string($user
$users_email = mysql_real_escape_string($user
= mysql_real_escape_string($user
`Inquiries`.`Inquiries` (`IDKEY`, `FNAME`, `LNAME`, `EMAIL`,
`COMMENTS`, `DATE`) VALUES (NULL, ‘$users_firstname’,
‘$users_lastname’, ‘$users_email’, ‘$users_comments’,
CURRENT_TIMESTAMP);”; mysql_query($query); echo “<h2>Thank you for
your Comment!</h2>”; mysql_close($con); } ?>
also – what does the $users mean before the form id fields? do i need to change that to something else?
Thanks!
Hello amit,
I would have your code echo out the query to ensure they are getting populated. Also make sure you error check the query as well for any information it may be putting out.
Kindest Regards,
Scott M
im trying to add data to my database bt its not. kindly assist guys
<?php
require_once(‘connect.php’);
//receive values from form and assign them to variable
$school_id = $_POST[‘school_id’];
$sch_name = $_POST[‘sch_name’];
$team = $_POST[‘team’];
$amount = $_POST[‘amount’];
$telephone = $_POST[‘telephone’];
$year = $_POST[‘year’];
//execute insert statement
$mydata =”insert into registration (school_id,sch_name,team,amount,telephone,year)
values(‘$school_id’,’$sch_name’,’$team’,’$amount’,’$telephone’,’$year’)”;
if(!mysql_query($mydata))
{
echo “Record not added into the table”;
}
else
echo “Recorded added successfully!”;
mysql_close($mydata);
?>
Could you clarify what exactly happens when you attempt to run your script?
Can’t figure out what’s going on here. It’s a singe page, single topic setup. I’ve embeded the code for manage_comments.php straight into index.html – and it all seems to work, except for actually putting the comment into the database. It does say “Thank you for your Comment! – but there’s no database entry. Any ideas?
<?
if( $_POST )
{
$con = mysql_connect(“localhost”,”xxxxx”,”yyyyy”);
if (!$con)
{
die(‘Could not connect: ‘ . mysql_error());
}
mysql_select_db(“databasename”, $con);
$users_comment = $_POST[‘comment’];
$users_comment = mysql_real_escape_string($users_comment);
$query = “
INSERT INTO `databasename`.`comments` (`id`,`timestamp`, `comment`) VALUES (DEFAULT, CURRENT_TIMESTAMP, ‘$users_comment’);”;
mysql_query($query);
echo “<h2>Thank you for your Comment!</h2>”;
mysql_close($con);
}
?>
<?php include(‘formcode.php’); ?>
The database has 3 columns. ID (whcih auto-increments with DEFAULT), timestamp, and the actualy comment. Nothing gets populated, no error message. Just the flash on the screen saying “Thank you for your Comment”.
Looking over your code, it looks fine. To further debug the issue, I recommend that you echo out the $query variable that you have set and take a look at it. If it looks fine, try to run it through PHPMyAdmin and see what happens.
Hello, i have used this code and after pressing the submit button the query is executed and displays the message “Thank You For Your Comment ” but when i open my datatbase the data is not inserted into table, everything is correct but still data is not inserted…???? help??
Could you provide us with the code that is causing the issue?
COULD NOT CONNECT MYSQL_ERROR
HELP ME BRO
<?php
if(isset($_POST[‘form’]))
$con=mysql_connect(“localhost”,”root”,””);
$db=”dum”;
if
(!$con)
{
die(“could not connect:mysql_error()”);
}
if (mysql_query(“CREATE DATABASE dum;$con”))
{
echo”your Database created which name is:dum”;
}
else
{
echo”Error creating database:”.mysql_error();
}
//create table
if(!$con)
mysql_select_db(“dum”,$con);
{
die(‘could not connect:’.mysql_error());
}
if(! get_magic_quotes_gpc() )
$sql=”CREATE TABLE
(
name VARCHAR (50);
destination VACHAR (30);
sal int(10);
qualificaqtion VARCHAR (35);
)”;
mysql_query($dum,$con);
echo”your table created which is as follows”;
//insert data
{
$name=$_POST[‘name’];
$destinatiion=$_POST[‘destination’];
$sal=$_POST[‘sal’];
$qualification=$_POST[‘qualification’];
$sql=”INSERT INTO $gum(id,name,destination,sal,qualification)
VALUES(‘$name’,’$destionation’,’$sal’,’$qualification’)”;
mysql_query($query);
if(! $retval )
{
die(‘Could not enter data: ‘ . mysql_error());
}
echo “Entered data successfully\n”;
}
mysql_close($con)
?>
<table border=”1″>
<tr>
<td align=”center”>Form Input Employees data</td>
</tr>
<tr>
<td>
<table>
<form method=”post”actio=”post”>
<tr>
<td>Name</td>
<td><input type=”text”name=”name”size=”50″>
</td>
</tr>
<tr>
<td>Destination</td>
<td><input type=”text”name=”Destination”size=”30″>
</td>
</tr>
<tr>
<td>sal</td>
<td><input type=”text”name=”sal”size=”10″>
</td>
</tr>
<tr>
<td>Qualification</td>
<td><input type=”text”name=”Qualification”size=”35″ >
</td>
</tr>
<tr>
<td></td>
<td align=”right”><input type=”submit”
name=”submit”value=”sent” ></td>
</tr>
</table>
</td>
</tr>
</table>
</body>
</html><?php
if(isset($_POST[‘form’]))
$con=mysql_connect(“localhost”,”root”,””);
$db=”dum”;
if
(!$con)
{
die(“could not connect:mysql_error()”);
}
if (mysql_query(“CREATE DATABASE dum;$con”))
{
echo”your Database created which name is:dum”;
}
else
{
echo”Error creating database:”.mysql_error();
}
//create table
if(!$con)
mysql_select_db(“dum”,$con);
{
die(‘could not connect:’.mysql_error());
}
if(! get_magic_quotes_gpc() )
$sql=”CREATE TABLE
(
name VARCHAR (50);
destination VACHAR (30);
sal int(10);
qualificaqtion VARCHAR (35);
)”;
mysql_query($dum,$con);
echo”your table created which is as follows”;
//insert data
{
$name=$_POST[‘name’];
$destinatiion=$_POST[‘destination’];
$sal=$_POST[‘sal’];
$qualification=$_POST[‘qualification’];
$sql=”INSERT INTO $gum(id,name,destination,sal,qualification)
VALUES(‘$name’,’$destionation’,’$sal’,’$qualification’)”;
mysql_query($query);
if(! $retval )
{
die(‘Could not enter data: ‘ . mysql_error());
}
echo “Entered data successfully\n”;
}
mysql_close($con)
?>
<table border=”1″>
<tr>
<td align=”center”>Form Input Employees data</td>
</tr>
<tr>
<td>
<table>
<form method=”post”actio=”post”>
<tr>
<td>Name</td>
<td><input type=”text”name=”name”size=”50″>
</td>
</tr>
<tr>
<td>Destination</td>
<td><input type=”text”name=”Destination”size=”30″>
</td>
</tr>
<tr>
<td>sal</td>
<td><input type=”text”name=”sal”size=”10″>
</td>
</tr>
<tr>
<td>Qualification</td>
<td><input type=”text”name=”Qualification”size=”35″ >
</td>
</tr>
<tr>
<td></td>
<td align=”right”><input type=”submit”
name=”submit”value=”sent” ></td>
</tr>
</table>
</td>
</tr>
</table>
</body>
</html>
Hello Shams,
Running the code you provided, the first line evaluates as False. This means it will not run the second line of code, which is where your connection should set up. Since the connection is never set up nor connected it fails the check and gives the error code you see on the screen.
To learn more about this please check our article on Connecting to a Database using PHP.
Kindest Regards,
Scott M
invalid article id
This error i get all time.
Hello Amir,
It looks like you’ve got an extra entry in your MySQL connect line, here is yours:
$con = mysql_connect("mysql1.000webhost.com","db_user","db_pass","comments");
While the original mentioned in this article is:
It appears the code that you have pasted in the comment above is not the full PHP script detailed in this guide. As you seem to be missing this section of the code dealing with throwing that error invalid article id:
If you’ve removed this line, the script should still function and place a comment in the database. You might want to double-check to ensure that you’ve setup a database to handle form data correctly, and also that you properly create a HTML form to get user comments.
– Jacob
Thank your for this but i get always invalid article.
I tryed delete articlied process but still can not insert a new comment in a database.
This is mine php code.
This code should appropriately insert data into a database. What is the specific error you are getting?