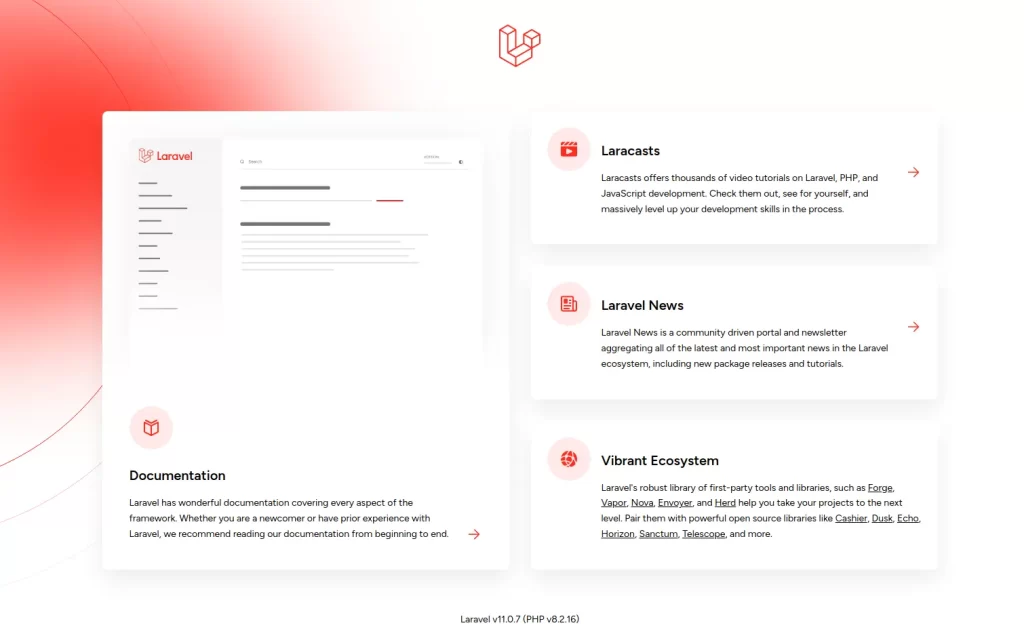
Laravel 11, launched on March 12, 2024, marks a significant update to the Laravel framework, introducing a range of new features and improvements aimed at enhancing the development experience and application performance. With a focus on efficiency, security, and real-time web application development, Laravel 11 brings forward several key enhancements and introduces Laravel Reverb, a new WebSocket server for real-time communication.
- Laravel Support Policy
- PHP 8.2 Requirement
- Streamlined Directory Structure
- Eager Load Limit
- Model Casts as Methods
- Laravel Reverb
- New Artisan Commands
- Health Route and APP_KEY Rotation
- Install Laravel 11
- How to Upgrade to Laravel 11
- Conclusion
Laravel Support Policy
Laravel’s support policy ensures that developers receive timely updates, bug fixes, and security patches for their applications. According to the policy, Laravel provides 18 months of bug fix support and 2 years of security fix support for each major release. This structured approach to version support enables developers to plan their upgrade paths effectively, ensuring their applications remain secure and up-to-date.
Version | Supported PHP | Release Date | Bug Fixes Until | Security Fixes Until |
---|---|---|---|---|
Laravel 9* | 8.0 – 8.2 | February 8, 2022 | August 8, 2023 | February 6, 2024 |
Laravel 10 | 8.1 – 8.3 | February 14, 2023 | August 6, 2024 | February 4, 2025 |
Laravel 11 | 8.2 – 8.3 | March 12, 2024 | September 3, 2025 | March 12, 2026 |
Laravel 12 | 8.2+ | Q1 2025 | Q3 2026 | Q1 2027 |
PHP 8.2 Requirement for Laravel 11
With the release of Laravel 11, a significant shift has been made in the PHP version requirement. Laravel 11 mandates PHP 8.2 as the minimum version, aligning the framework with the latest features, performance improvements, and security enhancements offered by PHP 8.2. This decision underscores Laravel’s commitment to leveraging the most current and robust technologies available, ensuring developers can build highly efficient, secure, and innovative applications.
PHP 8.2 introduces numerous new features and optimizations that can significantly benefit Laravel applications. These include enhancements to object-oriented programming, new types and attributes that encourage better coding practices, and performance improvements that can lead to faster application execution. By requiring PHP 8.2, Laravel ensures that all applications built on this version will inherently take advantage of these improvements, leading to more reliable and performant web solutions.
Developers planning to upgrade to Laravel 11 or start new projects with it must ensure their server environments are updated to PHP 8.2. This update not only facilitates compatibility with Laravel 11 but also positions applications to benefit from PHP’s active support, including security fixes and performance optimizations.
Streamlined Directory Structure
Laravel 11 simplifies its directory structure, reducing the initial complexity for developers. Notably, Kernel.php
files have been removed, and middleware can now be directly added in the bootstrap/app.php
file.
<?php
use Illuminate\Foundation\Application;
use Illuminate\Foundation\Configuration\Exceptions;
use Illuminate\Foundation\Configuration\Middleware;
return Application::configure(basePath: dirname(__DIR__))
->withRouting(
web: __DIR__.'/../routes/web.php',
commands: __DIR__.'/../routes/console.php',
health: '/up',
)
->withMiddleware(function (Middleware $middleware) {
//
})
->withExceptions(function (Exceptions $exceptions) {
//
})->create();
The config
directory has been slimmed down by removing some of the files that were previously included in Laravel 10 such as:
config/broadcasting.php
config/cors.php
config/hashing.php
config/sanctum.php
config/view.php
The routes
directory has also been cleaned up a bit by removing:
routes/api.php
routes/channel.php
routes/console.php
Eager Load Limit
The introduction of the eager load limit feature allows developers to efficiently query related models without overloading the system with unnecessary data. This feature optimizes the data loading process, making it possible to limit the number of related records loaded, thus improving application performance. In previous versions of Laravel you needed to install a separate package for eager load limit.
class User extends Model
{
public function posts()
{
return $this->hasMany(Post::class);
}
}
class Post extends Model
{
// ...
}
$users = User::with(['posts' => function ($query) {
$query->latest()->limit(10);
}])->get();
Model Casts as Methods
Laravel 11 allows defining casts as methods within model classes, offering more flexibility in how data is cast when retrieved from or stored in the database. This method-based approach provides a clearer and more dynamic way to handle data casting, enhancing the overall code readability and maintainability.
use App\Enums\UserOption;
use Illuminate\Database\Eloquent\Casts\AsEnumCollection;
// ...
/**
* Get the attributes that should be cast.
*
* @return array<string, string>
*/
protected function casts(): array
{
return [
'email_verified_at' => 'datetime',
'password' => 'hashed',
'options' => AsEnumCollection::of(UserOption::class),
];
}
For comparison, in Laravel 10 this model cast would be defined using the $cast
array property but you would not be able to call static methods.
protected $casts = [
'options' => AsEnumCollection::class.':'.UserOption::class,
];
Laravel Reverb: Real-Time Communication
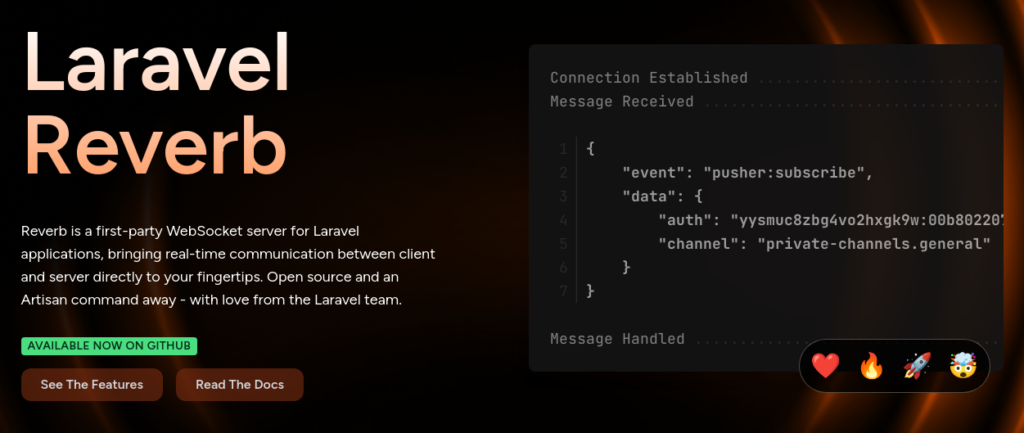
Laravel Reverb stands out as one of the most exciting additions in Laravel 11, offering a first-party WebSocket server that facilitates real-time communication between the client and server. Reverb is designed for high performance, capable of supporting thousands of connections simultaneously without the inefficiencies of HTTP polling. It integrates seamlessly with Laravel’s existing broadcasting capabilities and is built for scalability, with support for horizontal scaling using Redis. This makes it a robust solution for developers looking to incorporate real-time features into their applications, such as live chat, notifications, and dynamic content updates.
Reverb is optimized for speed and integrates smoothly with Laravel Forge for deployment, offering built-in monitoring support with Pulse. Its use of the Pusher protocol ensures compatibility with Laravel broadcasting and Laravel Echo, making it easier to develop engaging and interactive web applications.
Practical Implementation and Scaling
Implementing Laravel Reverb involves installing the package, configuring it according to your project’s requirements, and integrating its optimizations to enhance your application. Testing and validating these integrations are crucial to ensuring that the real-time features perform as expected. Monitoring application performance post-deployment is also recommended to identify and address any potential bottlenecks.
New Artisan Commands
Laravel 11’s new Artisan commands are a boon for developer productivity, significantly reducing the boilerplate code required when adding new components to an application. With commands for generating classes, enums, interfaces, and traits, developers can now scaffold new parts of their application with minimal effort. These commands are designed to streamline the development process, allowing for quicker setup and structuring of application logic and data representations. By using these commands, developers can adhere to best practices in code organization and maintainability, ensuring that their codebase remains clean and well-structured.
For example, the introduction of php artisan make:enum
simplifies the creation of enums, which are useful for defining a set of named constants. Enums can make your code more readable and less error-prone by limiting the values a variable can have. Similarly, the php artisan make:interface
and php artisan make:trait
commands facilitate the adoption of SOLID principles by promoting the use of interfaces for defining contracts within an application and traits for sharing methods across classes.
Health Route and APP_KEY Rotation
The /up
health route and the APP_KEY rotation feature are critical for maintaining the security and reliability of Laravel applications. The health route provides a simple way to verify that your application is running and responsive, making it invaluable for uptime monitoring and alerting systems. By integrating this route, developers can easily set up health checks that monitor the application’s status, quickly detecting and responding to any downtime.
The APP_KEY rotation feature addresses a common security concern by allowing for the secure rotation of the application’s encryption key without risking data loss. In previous versions of Laravel, changing the APP_KEY
could result in inaccessible data due to the encryption dependency on this key. Laravel 11 introduces a more flexible approach, allowing old data to be decrypted with previous keys specified in the APP_PREVIOUS_KEYS
environment variable while encrypting new data with the current key. This feature is essential for maintaining high-security standards, as regularly rotating keys can protect against certain types of cryptographic attacks without the drawback of losing access to encrypted data.
Install Laravel 11
If you are looking to set up a new Laravel 11 installation, you would just follow the normal procedures for installing Laravel manually or via Softaculous.
How to Upgrade to Laravel 11
There are two routes that you can take to upgrade to Laravel 11. You can manually upgrade by following Laravel’s Upgrade Guide or pay for Laravel Shift to automate the upgrade process.
Conclusion
Together, these enhancements in Laravel 11 underscore the framework’s commitment to facilitating efficient development practices while ensuring the security and resilience of applications. By incorporating these features, Laravel continues to offer a robust foundation for building and maintaining modern PHP applications, keeping pace with the evolving needs of developers and the industry at large.
Boost your Laravel apps with our specialized Laravel Hosting. Experience faster speeds for your Laravel applications and websites thanks to NVMe storage, server protection, dedicated resources, and optimization tools.
99.99% Uptime
Free SSL
Dedicated IP Address
Developer Tools